
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Defining Functions in Category: PHPlevel2 by amit
🕙 Posted on 2023-06-11 at 23:05:33 Read in Hindi ...
Custom Functions
So far, you have learned about defining variable names and constant names, different data-types, type-casting, operators, reserved keywords, control structures, and built-in (pre-defined) functions provided by the PHP developers in previous pages. These pre-defined functions are constructs (for example, echo
, print
), built-in functions (for example, var_dump()
, print_r()
, array()
, unset()
, Math Functions, String Functions, Array Functions, Variable Handling Functions), etc.
Functions are block of codes, used to perform specific task.
A function is a block of codes, which is whenever invoked, performs execution of those codes, in specific manner. You can call a function as many times as you need by just placing the name of the function (as you have seen in previous pages in case of pre-defined functions). However, there are some rules to define a function and to call that function in various manners.
Naming of Custom Functions
Unlike variable names and constant names, function names are case-insensitive. A function name is defined with the help of function
(reserved) keyword. A function name must always start with an English (roman) alphabetical letter (A-Z a-z) or underscore, followed by any number of letters, numbers, or underscores.
The function name must not contain any special character, including - (minus sign). A function name must be followed by ()
opening and closing parentheses, and thereafter one or more statements can be placed inside {}
opening and closing curly braces. Any valid PHP code may appear inside a function, even other functions and class definitions.
<?php
// syntax to write a custom function
function
my_function() {
echo 'Hello<br />';
}
my_function(); // Calling the function for first time
my_function(); // Calling the function for second time
my_function(); // Calling the function for third time
my_function(); // Calling the function for fourth time?>
Hello
Hello
Hello
A function can be rigid (as described in above example) or flexible. Generally, a custom function is not defined as shown above. The above code is to only describe the simplest illustration of a custom function. That is, the function name, my_function() defined above, cannot be used dynamically. Though ERROR may not be displayed as OUTPUT from following codes, these two are not correct syntaxes.
<?php echo my_function(); // same as: echo echo 'Hello<br />'; ?>
<?php print my_function(); // same as: print echo 'Hello<br />'; ?>
A function will be flexible when the output from such function name can be used as data (some operation can be performed on the data received). A function name always returns a value. In the above example, after execution of codes inside {}
curly braces, my_function() will return NULL
.
<?php var_dump( my_function() ); // output is as follows: ?>
NULL
<?php
function
myFunction() {
// No code inside the function, will also output NULL
}
var_dump( myFunction() ); // outputs NULL?>
return
keyword
The return (reserved) keyword is used to get the data (value) from the custom function, as output. The return
keyword inside a block {}
curly braces stops the processing of further codes, and outputs the value (from the literal or variable/return
keyword is used outside the {}
curly braces, that is, in any PHP file, then the codes below it will not be executed.
<?php
echo 'Hello<br />'; // outputs Hello
return
; // stops the processing of following code
echo 'Welcome<br />'; // Nothing will be OUTPUT?>
Caution
: You have to delete the above lines of codes from you PHP file, for example, phptest.php to get results from other examples.
<?php
// using return keyword inside a custom function
function
new_function() {
return
'Hello';
}
var_dump( new_function() );?>
As you can see in above example that the value returned from function new_function() is of string data-type. Now you can dynamically use the OUTPUT from the above example, in various places as per your need.
<?php
echo gettype
( new_function() ); // outputs string
echo '<br />';
$my_var = new_function();
echo strlen
( $my_var ); // outputs 5?>
PHP does not support function overloading, nor is it possible to undefine or redefine previously declared functions. In ONE request-response cycle, only one function name must be defined. As a function name is case-insensitive, you should use different names for different functions declared in ONE request-response cycle.
Functions are global in nature
A function name can be called before (at the top) defining it (that is, afterwards below calling of that function name, it can be defined). All functions and classes in PHP have the global
scope − they can be called outside a function, even if they were defined inside and vice versa. You will learn about global
and local
scopes in later pages. See the following example:
<?php
var_dump( foo() ); // a function name is global, therefore, can be called anywhere, except in two conditions.
function
foo() {
return
'Hello';
}?>
Calling an undefined function will throw ERROR.
You can call all functions from anywhere, except in two cases (1. conditional functions, and 2. variable functions). However, you must note that when a function name is not defined, ERROR will be shown as OUTPUT.
<?php
var_dump( not_defined_function() ); // outputs ERROR as shown below:?>
Fatal error: Uncaught Error: Call to undefined function not_defined_function() in C:\xampp\
There are certain cases in which you should be careful, as PHP interpreter processes the codes in a file from top to bottom, line-by-line. That is, when you write codes in any CODE EDITOR (e.g. VSCode Editor), the line 1 will be processed first, then line 2, line 3, and so on...
Though you can write a custom function inside another custom function, it is not advisable, and you should avoid such circumstances as possible. See the following example:
<?php
function
coo() {
echo 'Hello from Outside coo()<br />';
function
doo() {
echo 'Hello from doo()<br />';
function
coo() {
// codes inside this internal function can not be processed.
echo 'Hello from Inside coo()<br />';
}
}
}
// doo(); // outputs ERROR as shown below, when you uncomment this line
coo(); // outputs Hello from Outside coo()?>
Fatal error: Uncaught Error: Call to undefined function doo() in C:\xampp\
As soon as only coo() function is called, no ERROR is shown. This is called as short-circuit of code execution, that is, when any function is not called (not invoked), no codes inside it will be executed. When the doo() function is called before calling of coo() function, an ERROR (of undefined function) will be shown as shown above.
<?php doo(); // outputs ERROR as shown below along with Hello from doo() ?>
Fatal error: Cannot redeclare coo() (previously declared in C:\xampp\
You can call the coo() and doo() functions before defining them. And if you remove (delete) the coo(){} function (line 8, 9 and 10 of above example) defined inside the doo(){}, NO ERROR (of previously declared function) will be shown.
It is possible to call recursive functions in PHP. Recursive function/
You will learn more about functions in next pages.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
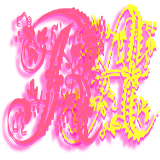
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇