
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Checking Data Types in Category: PHP by amit
🕙 Posted on 2023-05-24 at 21:01:29 Read in Hindi ...
Type Checking − Variable handling Functions
Though var_dump()
function tells you everything about a literal, or a variable/
gettype()
<?php echo gettype
( false ); // outputs boolean ?><br />
<?php echo gettype
( PHP_INT_MAX ); // outputs integer ?><br />
<?php echo gettype
( 12.56 ); // outputs double ?><br />
<?php echo gettype
( "true" ); // outputs string ?><br />
<?php echo gettype
( [ null ] ); // outputs array ?><br />
<?php echo gettype
( NULL ); // outputs NULL ?><br />
You can see in above examples that, gettype()
function outputs data-type of a literal or a variable/
<?php var_dump( gettype
( false ) ); // outputs string(7) "boolean" ?><br />
<?php var_dump( gettype
( PHP_INT_MAX ) ); // outputs string(7) "integer" ?><br />
<?php var_dump( gettype
( 12.56 ) ); // outputs string(6) "double" ?><br />
<?php var_dump( gettype
( "true" ) ); // outputs string(6) "string" ?><br />
<?php var_dump( gettype
( [ null ] ) ); // outputs string(5) "array" ?><br />
<?php var_dump( gettype
( NULL ) ); // outputs string(4) "NULL" ?><br />
There are other PHP pre-defined functions which can directly check the data-type of a literal or a variable/true
. When the literal or a variable/false
.
isset()
You have already seen the isset()
function in previous page, which outputs true
when the variable/NULL
), the output is false
.
<?php var_dump( isset
( $not_var ) ); // variable $not_var has not been defined, and output is bool(false) ?><br />
<?php $my_var; // No value has been assigned to variable $my_var
var_dump( isset
( $my_var ) ); // outputs bool(false) ?><br />
<?php $new_var = null
; // NULL represents no value.
var_dump( isset
( $new_var ) ); // outputs bool(false) ?><br />
<?php $array_var = array()
; // empty array literal has been assigned to variable $array_var
var_dump( isset
( $array_var ) ); // outputs bool(true) ?><br />
<?php $string_var = ""
; // empty string literal has been assigned to variable $string_var
var_dump( isset
( $string_var ) ); // outputs bool(true) ?><br />
<?php $bool_var = false
; // false or true can be assigned to variable $bool_var
var_dump( isset
( $bool_var ) ); // outputs bool(true) ?><br />
The isset()
function only checks the variable/""
(empty string), []
(empty array), NULL
, etc. In following example, each code outputs the same ERROR as shown below.
<?php var_dump( isset
( "" ) ); // outputs ERROR ?><br />
<?php var_dump( isset
( [] ) ); // outputs ERROR ?><br />
<?php var_dump( isset
( NULL ) ); // outputs ERROR ?><br />
<?php var_dump( isset
( false ) ); // outputs ERROR ?><br />
Fatal error: Cannot use isset() on the result of an expression (you can use "null !== expression" instead) in C:\xampp\
is_bool()
is_bool()
function checks the literal, a variable/true
or false
or output from that expression is one of these boolean values, then output will be bool(true). You should note that is_bool()
function is different from type-casting, and any integer, float, string, array evaluated as true or false are not BOOLEAN values, and therefore, output will bool(false). See the examples below:
<?php var_dump( is_bool
( false ) ); // outputs bool(true) ?><br />
<?php var_dump( is_bool
( true ) ); // outputs bool(true) ?><br />
<?php var_dump( is_bool
( 0 ) ); // outputs bool(false) ?><br />
<?php var_dump( is_bool
( 1 ) ); // outputs bool(false) ?><br />
<?php var_dump( is_bool
( "" ) ); // outputs bool(false) ?><br />
<?php var_dump( is_bool
( [] ) ); // outputs bool(false) ?><br />
is_int(), is_integer(), or is_long()
is_integer()
and is_long()
functions are alias of is_int()
function. All these three function check whether a literal or a variable/0
(zero) or 1
or other integer value, will output false, when checking with these three functions.
<?php var_dump( is_int
( "12" ) ); // outputs bool(false) ?><br />
<?php var_dump( is_int
( intdiv
( PHP_INT_MAX, PHP_INT_MIN ) ) ); // outputs bool(true) ?><br />
intdiv()
is a Math function, similar to fdiv()
, but it outputs an integer value when operation is successful. Following code will output ERROR as shown below.
<?php var_dump( is_int
( intdiv
( PHP_INT_MIN, -1 ) ) ); // outputs ERROR ?><br />
Fatal error: Uncaught ArithmeticError: Division of PHP_INT_MIN by -1 is not an integer in C:\xampp\
<?php var_dump( is_int
( intdiv
( 1, 0 ) ) ); // outputs ERROR ?><br />
Fatal error: Uncaught DivisionByZeroError: Division by zero in C:\xampp\
is_float(), is_double(), or is_real()
is_double()
and is_real()
functions are alias of is_float()
function. All these three functions check whether a literal or a variable/fdiv()
and fmod()
are Math functions, which output floating point numbers.
<?php var_dump( is_float
( M_PI ) ); // outputs bool(true) ?><br />
<?php var_dump( is_float
( NAN ) ); // outputs bool(true) ?><br />
<?php var_dump( is_float
( fdiv
( 1, 0 ) ) ); // outputs bool(true) ?><br />
<?php var_dump( is_float
( fmod
( 21, 0 ) ) ); // outputs bool(true) ?><br />
More Variable Handling Functions, and Checking Values of Variables, are described in next page.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
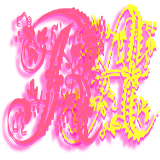
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇