
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Mathematical Operations in Category: PHP by amit
🕙 Posted on 2023-05-06 at 19:22:19 Read in Hindi ...
NULL
, INF
(infinity) NAN
(not a number), and Math Functions
NULL
or null
is a pre-defined literal of special type, which is generally used to remove (some) value from a variable name. In previous page, you have seen how unset()
function not only remove the value in a variable, but also destroys that variable. This will result in a situation, where that variable name has not been existed. This leads to cause certain ERROR.
However, using null
does not result in such type of ERROR. You must also note that any variable name which does not exist, whether it is undefined (not defined) or it is destroyed by unset()
function, the value shall be null
for that variable name. null
represents a variable or constant with no value. You can assign a constant name with null
value, but it cannot be changed later!
<?php
echo null
; // outputs nothing
echo '<br />'; echo print( null
); // outputs 1 because of print() function
echo '<br />'; echo (int) null
; // outputs 0 (zero)
echo '<br />'; echo (float) null
; // outputs 0 (zero)
echo '<br />'; echo (bool) null
; // outputs nothing (false)?>
Type casting a variable name or constant name to null
has been DEPRECATED as of PHP 7.2.0, and REMOVED as of PHP 8.0.0. You should assign to that variable or constant name to null
manually. You should carefully examine the version of PHP installed on your local computer or remote server.
Infinity INF
When any number is divided by 0
(zero), the result is infinity. But, computer engineers and programmers have not allowed us (general public, web-developers, etc.) to directly divide any number by 0
(zero). This will cause ERROR, because no number can represent infinity, either negative or positive! Different programming languages uses different keywords to represent infinity.
NAN
NAN
is a reserved keyword, generally used to check whether any literal is numeric or not numeric (that is, not a number). You should refer the official documentation of each programming languages, for these reserved keywords, that is, null
is case-insensitive in PHP, but INF
and NAN
both are case-sensitive in PHP. (null, Infinity and NaN are reserved keywords in javascript, which are case-sensitive.)
<?php
var_dump( NAN
); // case-sensitive
echo '<br />'; var_dump( INF
); // case-sensitive
echo '<br />'; var_dump( 2/0 ); // displays ERROR as show below?>
float(INF)
Fatal error: Uncaught DivisionBy
Math Functions
There are certain built-in functions in each programming languages, which are necessary to run our script/
pi()
or M_PI
You have seen in previous page, that we can get value of PI dividing 22 by 7, however, actual value is different and can be obtained by the Math function, pi()
or its equivalent constant M_PI
. The pre-defined Math function, pi()
takes no argument, that is no value must be placed inside parentheses.
<?php
var_dump( pi()
); // float(3.141592
echo '<br />'; var_dump( M_PI
); // float(3.141592
echo '<br />'; var_dump( 21.991148575/7 ); // float(3.141592?>
fdiv()
and fmod()
You have seen /
(division operator) and %
(modulus operator) which are generally used by programmers. However, when any floating point number is divided by another floating point number or a integer (as described in above example), or vice-versa, then results may be unexpected. Therefore, these two pre-defined Math function are used to get the correct results for such operations:
<?php
echo fdiv
(22, -7); // -3.142857
echo '<br />'; echo fdiv
(-22, 0); // -INF
echo '<br />'; echo fmod
(22, -7); // 1 (sign of remainder is decided by first argument, which is divided by second argument in case of modulus operation)
echo '<br />'; echo fmod
(-22, -7); // -1
echo '<br />'; echo fmod
(-22, 7); // -1?>
abs() , pow() , sqrt() and rand() functions
abs()
is absolute function (it converts negative numbers to positive numbers), pow()
is power of function (equivalent to exponential operator, but generally used for floating point numbers). pow() function takes two arguments separated by , (comma) that is second number is exponential power of first number. sqrt()
is square root function. When negative number is placed inside parentheses of sqrt() function, the output is NAN (not a number). rand()
is random output function. You must either put no argument inside rand() function, or place two arguments separated by , (comma) between which any random value is returned.
<?php
echo abs
( -25.6 ); // 25.6
echo '<br />'; echo pow
( 3.2, 4 ); // 3.2*3.2*3.2*3.2* that is, 104.8576
echo '<br />'; echo sqrt
( -8.1 ); // outputs NAN for negative numbers
echo '<br />'; echo sqrt
( 8.1 ); // outputs 2.84604
echo '<br />'; echo rand
(); // outputs any random number (of maximum 10 digits)
echo '<br />'; echo rand
( -10, 10 ); // outputs numbers between -10 (minimum) and 10 (maximum)?>
round() , ceil() and floor() functions
round()
function rounds the fractional (floating point) number to certain limit (after decimal point) when second argument is passed, otherwise it will rounds that number to integer value. General rule of mathematics is applied to round a fractional value to certain limit. That is, if last digit (on right side) after decimal point is more than 4 (four), then the digit to the left of it, is increased by 1, and so on...
<?php
echo round
( 2.846049
echo '<br />'; echo round
( 3.141592
echo '<br />'; echo round
( pi()
, 2 ); // 3.14?>
In the above example, if last digit of 2.846049
ceil()
function rounds the fraction to up (value before decimal point is increased by 1), and floor()
function rounds the fractional value to down (value before decimal point is decreased by 1). ceil()
and floor()
functions take only one argument, that is, only one floating point value must be placed within parentheses, and output in both cases, is an integer value, but of float type.
<?php
var_dump( ceil
( 4.345 ) ); // outputs float(5) but round() function returns 4
echo '<br />'; var_dump( floor
( 4.567 ) ); // outputs float(4) but round() function returns 5?>
min() , max(), log() and other Math functions:
min()
and max()
are Math functions, both of which are used to find minimum and maximum value stored in different items of an array. Array is a compound data-type, which will be explained in next pages.
<?php
echo max
(2, 3, 1, 6, 7); // outputs 7
echo '<br />'; echo max
( array(2, 4, 5) ); // outputs 5
echo '<br />'; echo min
(2, 3, 1, 6, 7); // outputs 1
echo '<br />'; echo min
( array(2, 4, 5) ); // outputs 2
echo '<br />'; echo log
( -1 ); // outputs NAN
echo '<br />'; echo log
( 1 ); // outputs 0 (zero)?>
Other Math functions are specifically used for specialized cases, such as finding value of sine θ, cosine θ, tan θ, etc. bindec()
(binary to decimal), decbin()
(decimal to binary), dechex()
(decimal to hexadecimal), hexdec()
(hexadecimal to decimal), decoct()
(decimal to octal), octdec()
(octal to decimal), deg2rad()
(degree to radian), rad2deg()
(radian to degree), etc. are self-explanatory, that is these functions are used to convert one BASE system into another BASE system.
There are many other Math functions, which are used to check values and their data-types, and they return either true or false. These functions including other similar functions, to check other data-types, etc. will be explained in next pages.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
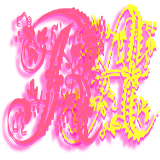
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇