
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Conditional Blocks in Category: Python by amit
🕙 Posted on 2023-07-03 at 20:21:56 Read in Hindi ...
Control Structures
Control structures are block of codes, which are used to perform specific tasks. Conditional statements, Loops, Functions and Classes are control structures. Till now, in Python tutorials, indentation is not used. However, writing control structures, you must be careful regarding indentation, and maintain levels of indentation in block of codes NESTED inside another block of code.
Conditional Statements
There are three types of conditional statements − (1) if
block, (2) if else
block, and (3) if elif else
block, which you can use in Python programs. All three are important, and syntax of these are described as follows.
In following three examples, you can see different types of conditional statements (control structures), which will perform certain task (that is, execute some block of codes) when the conditional expression is True
. In these three examples, only literal values of boolean data-type is provided to explain them in simple way.
You can write as many independent if
conditional block, which may be necessary in certain situations. Each if will check the conditional expression after it, and when it is evaluated as True
, then only, block of codes below it, within indentation shall be executed. The indentation may be of 1, 2, 3, or 4 blank (white-) spaces.
if
True:
print( "Condition is True, and block will be executed." )
In above code two indentation spaces have been provided in second line. Similarly, in following examples, after every conditional expression checking, that is, after the :
(colon) symbol, two indentation spaces are provided in next line.
The if else
block of code will execute only one set of statements after either of :
(colon) symbol. That is, when the conditional expression is True, the first set of statements (below if
) will be executed, and when the conditional expression is False, the second set of statements (below else
) will be executed.
In following example, you can see that both if
and else
keywords are without indentation, and codes below them are specifically written after two blank (white-) spaces. You have to provide same level of indentation for each of blocks, and you should not mismatch the indentation (blank spaces), otherwise the IndentationError may be displayed.
if
False:
print( "Condition is False, this statement will not be executed." )else
:
print( "Statement below the else will be executed, when condition is False." )
In PHP and JavaScript, to check many conditions, if elseif else or if else if else (a blank space between else if) conditional statements are used. But in Python, you should be careful, and only use if elif else
control structure. elif is a reserved keyword and not short (or abbreviated) form of else if or elseif. The functionality of these control structures are same in all three programming languages, and other C based programming languages.
In the following example, only one set of statements (inside either conditional block) will be executed. Herein below example, only one statement is written (to simply describe that how following example works,) in either if
, elif
, and else
block, though as many statements can be written after the :
(colon) symbol (from next line) within indentation.
You can add as many elif
conditions in between if
and else
blocks, but only one set of statements will be executed, when either any one condition is evaluated as True, or all conditions are evaluated as False, then the last set of statements after else
block will be executed. You need not to provide the else
block, and thence, when all conditions are evaluted as False, nothing in the if elif
block will be executed.
if
False:
print( "Condition is False, this statement will not be executed." )elif
True:
print( "Statement below elif will be executed, when if condition is False, and elif condition is True." )else
:
print( "Statement below the else will be executed, when above two conditions are False." )
In the following example, you can see that all three statements before the if elif else
block of codes, are without indentation. In these three statements, that is condition1 = False, condition2 = True, and condition3 = False, three variable names have been assigned with boolean literals. These statements can be conditional expressions, for example, 5 < 4, which is evaluated as False.
condition1 = False
condition2 = True
condition3 = True
# In the following if-elif-else block of codes,
# different indentation levels are color coded.
# Two blank spaces are colored yellow, and
# Four blank spaces are colored green.
if condition1:
elif condition2:
else:
With the help of these if
, if else
, and if elif else
blocks, you can create complex CLI Application, or WEB-Development App, etc. In the advanced section, you will learn to build sophisticated Astrology APP, which can be much more complex, than you have been learning in Basic Python Skills. In the following example, you will learn, how to determine whether a year is a leap year or not.
There is complex calculation, as shown below unlike in school, we have been taught that any year which is divisible by 4, is a leap year. This is how you find out that if a particular year is a leap year:
(1) on every year that is evenly divisible by 4, but
(2) that year is not evenly divisible by 100,
(3) and when that year is evenly divisible by 100, is also evenly divisible by 400.
In the following three examples, you can also see that different indentation levels are color-coded, that is two blank spaces are colored yellow, four blank spaces are colored green, and six blank spaces are colored cyan. To write above exercise that is, to determine a given number as leap year, you should first write following if else
block of codes.
if
year % 4 == 0:
else
:
Then, insert the first NESTED if else
block of codes as follows.
if
year % 4 == 0:
if
year % 100 == 0:
else
:
else
:
After that, you can insert another NESTED if else
block of codes as shown in below (complete) example.
year = int
( input( "Enter the year to check for leap year: " ) )if
year % 4 == 0:
if
year % 100 == 0:
if
year % 400 == 0:
else
:
else
:
else
:
Some years are shown below to explain you the concept of LEAP Year. When the first condition (image as a person, for example) is evaluated as True, then the year provided by the user will be a Leap Year, only when the NESTED condition (person's child) is evaluated as False. When the NESTED condition (child) is evaluated as False, then the another NESTED condition (person's grand-child) shall not be evaluated. But when the first NESTED condition (child) is evaluated as True, then that year will not be a Leap Year, except when the NESTED condition (grand-child) is also evaluated as True. When all three conditions are evaluated as True, then that year will be a Leap Year.
(2000%4==0) and (2000%100==0) and (2000%400==0) is a LEAP YEAR.
(2100%4==0) and (2100%100==0) and (2100%400==100) is NOT LEAP YEAR.
(1900%4==0) and (1900%100==0) and (1900%400==300) is NOT LEAP YEAR.
(2400%4==0) and (2400%100==0) and (2400%400==0) is a LEAP YEAR.
(2024%4==0) and (2024%100==24) and (2024%400 not necessary) is a LEAP YEAR.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
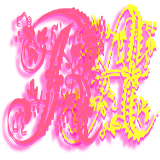
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇