
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Loops in Category: Python by amit
🕙 Posted on 2023-07-06 at 14:44:53 Read in Hindi ...
Loops in Python
A LOOP is a control structure, which on fulfilling of certain condition, repeats a block of codes. When that condition becomes False
, or break
statement is used inside the LOOP, then that loop stops executing the block of codes. There are two types of LOOPs − (a) Definite/
Unlike PHP and JavaScript, there are only two type of loops in Python − (1) while
and (2) for
. The while loop behaves like general loop in which you have to declare (i) initialization, (ii) conditional expression, and (iii) iteration. However, the for loop works only on collection of items or characters (sequences), and thus, it behaves like foreach loop in other programming languages, such as PHP and JavaScript.
while Loop
Following two examples are typical illustration of finite while
loop, where you have to (i) initialize the numeric data-type variable, (ii) place condition after the while
keyword, and (iii) after the :
(colon) symbol, in next line, inside the block of codes, iterate (either increment or decrement) the numeric data-type variable. When the condition becomes False, the while
loop exits out of the block.
num = 0 # initialization
while num < 10:
print( "Outside while loop: ", num )
num = 0 # initialization
while num > -10:
print( "Outside while loop: ", num )
There is no ++ or -- operators in Python, therefore, you should use either arithematic operators, or arithematic assignment operators. You can copy and save both of above examples in a file with .py extension, for example, new.py in your project folder, and then run/
C:\xampp\
0
1
2
3
4
5
6
7
8
9
Outside while loop: 10
0
-1
-2
-3
-4
-5
-6
-7
-8
-9
Outside while loop: -10
There are many ways in which you can do operations, such as finding squares ** 2, squre-root ** (1/2), cubes ** 3, or cube-root ** (1/3), etc. of each numbers.
num = 0
while num < 10:
print( "Outside while loop: ", num )
Many programmers also use else
keyword after the while block of codes, to execute/while
loop is evaluated as False. In WEB Developement, and other applications, you may encounter such situations, and therefore you can also use else
keyword after the while block. For example:
num = 0
while num > 10:
else:
print( "The statement in this line, has no connection with while loop." )
When you replace the > (greater than) operator with < (less than), the above example will output square-else
keyword thence also, outputs the result, when the condition is evaluated as False, at the end of the program. This is different from if else
or if elif else
block of codes, where only one set of statements (after either if, elif, or else), is the output (result).
C:\xampp\
The condition is False, therefore, num is 0
The statement in this line, has no connection with while loop.
C:\xampp\
1.0
1.41421356
1.73205080
2.0
2.2360679
2.4494897
2.6457513
2.8284271
3.0
3.16227766
The condition is False, therefore, num is 10
The statement in this line, has no connection with while loop.
Exhaustive Loop
In the following example, the condition is evaluated as True, and a NESTED if
block is inserted to break
the loop when condition will be equal to 3. In this example, the else
block (one or more lines of codes) will not be executed. The statement below the if
condition is indented four blank spaces. When you comment the if
condition, and the indented statement (break
) below it, the while loop will be exhausted completely.
num = 0
while num < 10:
break
else:
print( "The loop is broken by the break keyword." )
Infinite while Loop
Caution
: In each of above examples, the while
loop has been iterated either by increasing or decreasing the variable name, num initialized before it. You can see that in every block of codes below the while
condition, the statement num += 1 or num -= 1 has been placed before or after other statements.
When either you remove the iteration statement, that is, num += 1 or num -= 1, or in certain situations, the iteration statement is placed so that it cannot be executed; then the while
loop will beocme infinite loop. When you run/
You have to press Ctrl C or Cmd C keys simultaneously to terminate/
num = 0
while num < 10:
continue
# continue will skip all codes below it inside the block.
else:
print( "The loop is skipped by the continue keyword." ) # Press Ctrl C or Cmd C keys simultaneously.
Practical Uses of Indefinite Loops
In real world WEB Applications, the user (public) wants to do certain task repeatedly, and the programmer doesn't know, how many times, the codes should be executed. In such situations, indefinite or infinite loops are very useful, for example:
while True:
int
( input( "Please enter a number between 1 and 10. Thereafter, press 'Enter' or 'Return' key: " ) )
break
print( "You played the game. Bye!" )
In the above example, the while
condition is always evaluated as True. Hence, it is a infinite loop, in which the public (user) has been asked to input certain number. Remember that the input()
function always returns a string data-type. Therefore, to compare it with any numeric value, you must have to type-cast the returned value with int()
or float()
in-built functions. When the user will enter 5 and press Enter or Return key, the infinite while
loop shall be terminated.
for Loop
The for
loop only works on sequence data-types, such as string, list, tuple, etc. In the following example, the string 'Hello' is a sequence of five letters, and the for
loop outputs each lettter in new line.
string = 'Hello'
for item in
string:
C:\xampp\
H
e
l
l
o
A list is the collection of items, enclosed within []
(square brackets). Herein below, a list of prime numbers are shown as example. You will learn more about different data-types and application of while
and for
loops in next pages.
for number in
[1, 2, 3, 5, 7, 11, 13, 17, 19]:
C:\xampp\
1
2
3
5
7
11
13
17
19
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
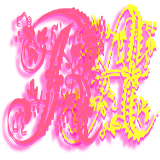
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇