
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
List Methods in Category: Python by amit
🕙 Posted on 2023-08-16 at 11:19:19 Read in Hindi ...
Change the Value of any item/ element of Mutable Data-Types
The value assigned to a variable in Python can be simple scalar data-type or simple sequence data-type, or collection of lists/
Example 1
fruits = ["Apple", "Banana", "Kiwi", "Mango", "Orange"]
print( fruits[1:4][0][1:4] ) # Outputs ana, Slicing a data-type outputs the same data-type that is list, which can be further sliced.
print( fruits[1][1:4] ) # Outputs ana, [1] extracts a string, which if extracted with [0], outputs B (one letter, cannot be further sliced.)
You can change the value of an item/
Example 2
nested_list = [1, 'a', [2, 3, 'b'], [4, [5, 6, 'c']], 7, 'd']
nested_list[1] = 'x'
nested_list[2][2] = 'y'
print( nested_list ) # The values of respective items are changed as shown below:
Example 3
Unlike other programming languages, you can only change the value of an item of a list, but you cannot add an item to a list (whether it is empty or not) by using following way. However, an item can be added to a list using pre-defined append()
, extend()
and insert()
methods (described below).
empty_list = []
empty_list[0] = "Hello" # Outputs IndexError: list assignment index out of range
empty_list[] = "World" # Outputs SyntaxError: invalid syntax (Comment above statement, to see this result.)
print( empty_list )
You can also delete a variable name with del
(reserved keyword), as it was never defined. But you cannot delete an item or a character from a immutable data-type, such as a tuple or a string. However, you can delete an item/
Example 4
my_list = [1, "Amit", object(), 3.14, True, (1, 2.3, 3), None]
print( my_list[2] ) # Outputs <object object at 0x00000112del
my_list[6] # Data-type of item is not important, but its parent must be mutable data-type.
print( my_list ) # Outputs [1, 'Amit', object(), 3.14, True, (1, 2.3, 3)]del
my_list[5][1] # Comment this line to see result of below statements.
print( my_list ) # Outputs ERROR as shown below, because the value of any item of a tuple cannot be changed.
Example 4 continued ...
del
my_list # The value of variable name, my_list is unset.
print( my_list ) # Outputs ERROR as shown below, because variable name, my_list is now undefined.
List Methods
You have already learned how to apply count()
and index()
methods, and len()
built-in function on list and tuple data-types in previous page. Also, you have learned about NESTED lists in last page. Help documentation of all list methods are described already in How to Get Help page. These concepts are important before you will learn about following list methods:
append(), extend() and insert()
Example 5
empty_list = []
empty_list.append
( 'Hello' )
print( empty_list ) # Outputs ['Hello']
Example 5 continued ...
empty_list.append
( [1, 2, 3] ) # add these two lines below Example 5
print( empty_list ) # Outputs ['Hello', [1, 2, 3]]
In above Example 5, you can see that the append()
method joins the variable name or literal inside its parentheses after the last item/extend()
method adds all items/
Example 5 continued ...
empty_list.extend
( [4, 5, 6] ) # add these two lines below Example 5
print( empty_list ) # Outputs ['Hello', [1, 2, 3], 4, 5, 6]
empty_list.extend
( 'World' ) # add these two lines below Example 5
print( empty_list ) # Outputs ['Hello', [1, 2, 3], 4, 5, 6, 'W', 'o', 'r', 'l', 'd']
Example 5 continued ...
empty_list.insert
( 5, [7, 8, 9] ) # add these two lines below Example 5
print( empty_list ) # Outputs ['Hello', [1, 2, 3], 4, 5, 6, [7, 8, 9], 'W', 'o', 'r', 'l', 'd']
The insert()
method requires the index number (integer) of the parent list, as the first argument where the second argument (string, list, etc.) can be appended. Other items/
Example 6
In the following example, you can see that only iterable data-type can be converted (type-casted) into list data-type with help of list()
built-in function. Many tutorials tell you that list() built-in function can create a list data-type. However, numbers (integers and floats), booleans (True, False
) and None
cannot be converted into list data-type, with list() built-in function. Dictionaries have keys and their respective values, which can only be converted into list data-type with help of dictionary methods (explained in next page).
iterable_string = '12345' # 'Hello' (characters will be split into individual items)
iterable_tuple = (1,2,3,4,5)
iterable_set = {1,2,3,4,5}
non_iterable = 12345
print( type( iterable_string ), list( iterable_string ) )
print( type( iterable_tuple ), list( iterable_tuple ) )
print( type( iterable_set ), list( iterable_set ) )
print( type( non_iterable ), list( non_iterable ) )
<class 'tuple'> [1, 2, 3, 4, 5]
<class 'set'> [1, 2, 3, 4, 5]
Traceback (most recent call last):
File "C:\xampp\
print( type( non_iterable ), list( non_iterable ) )
^^^^^^^^^^
TypeError: 'int' object is not iterable
remove(), clear(), pop()
You can see herein above that applying append()
, extend()
or insert()
method on list literal/remove()
, clear()
or pop()
method will delete an item, all items, or last item respectively, from the list literal, and the original list will be modified thereafter. Following examples will explain the same.
Example 7
The remove()
method will remove the first occurrence of object (that is, value of the literal/variable name) provided inside the parentheses. The integer value passed as the argument is not the index number of the parent list. The object passed as an argument to the remove()
method if not found in the parent list, then ValueError will be shown as output.
my_list = [1, 2, 3, 4, 5, 6]
my_list.remove
( 2 )
print( my_list ) # Outputs [1, 3, 4, 5, 6]
my_list.remove
( 'Hello' ) # Comment this line to continue with following example.
print( my_list ) # Outputs ERROR as shown below:
Traceback (most recent call last):
File "C:\xampp\
my_list.remove( 'Hello' )
ValueError: list.remove(x): x not in list
Example 7 continued ...
my_list.clear()
# argument is not needed for clear() method
print( my_list ) # Outputs [] (always returns an empty list, because all items are deleted.)
Example 8
The pop()
method will remove the item/pop()
method will remove the last item. If you assign this expression, that is my_list.pop() to a variable name, then the item removed is returned by the pop()
method.
my_list = [1, 2, 3, 4, 5, 6]
new_var = my_list.pop()
# last item will be removed.
print( my_list ) # Outputs [1, 2, 3, 4, 5]
print( new_var ) # Outputs 6
new_var = my_list.pop
( 3 ) # index number starts from 0 (zero)
print( my_list ) # Outputs [1, 2, 3, 5]
print( new_var ) # Outputs 4
copy(), reverse(), sort()
The copy()
method only assigns the list literal to another variable name. This can also be done with assignment operator. The reverse()
method also sorts the items/
However, the sort()
method sorts the items not on index number basis, but on the basis of values of those items. The sort() method also takes reverse = True as an argument which sorts those values in reverse order (not on index basis) (reverse = False is default value).
Example 9
my_list = [2, 5, 7, 4, 3, 1, 8, 9, 6]
new_list = my_list.copy()
print( my_list ) # Outputs [2, 5, 7, 4, 3, 1, 8, 9, 6]
print( new_list ) # Outputs [2, 5, 7, 4, 3, 1, 8, 9, 6]
Example 9 continued ...
my_list.reverse()
print( my_list ) # Outputs [6, 9, 8, 1, 3, 4, 7, 5, 2]
my_list.sort()
print( my_list ) # Outputs [1, 2, 3, 4, 5, 6, 7, 8, 9]
my_list.sort
( reverse=True )
print( my_list ) # Outputs [9, 8, 7, 6, 5, 4, 3, 2, 1]
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
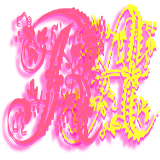
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇