
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
join(), replace(), concatenation in Category: Python by amit
🕙 Posted on 2023-07-24 at 08:59:51 Read in Hindi ...
Concatenation of Strings and Numbers
The two strings can simply be joined (concatenated) by using +
(concatenation operator) or +=
(concatenation assignment operator). You should also use " " (a string literal consisting of one blank space within a pair of double/
first_word = 'Good'
second_word = 'Morning'
full_word = first_word + second_word
print( full_word ) # concatenate two strings, outputs GoodMorning
word = 'Good'
word += 'Evening'
print( word ) # concatenate two strings, outputs GoodEvening
# The above example is explained herein below:
# You can place string literal directly after += operator.
first_word = 'Good'
second_word = 'Evening'
full_word = first_word # full_word = 'Good'
full_word += second_word # full_word = full_word + 'Evening'
print( full_word ) # concatenate two strings, outputs GoodEvening
print( 'Hello ' + 'World' ) # Outputs Hello World
print( 'Hello' + ' World' ) # Outputs Hello World
print( 'Hello' + ' ' + 'World' ) # Outputs Hello World
You can also join (concatenate) two or more strings or other data-type literals, with join()
, replace()
(which are explained below), and format()
methods, etc. (explained in next page). An integer or a float when concatenated (that is, added) to string literal, the numeric value must be first type-casted with str()
built-in function, otherwise ERROR will be shown.
A numeric string (that is, a number enclosed within a pair of double/int()
or float()
built-in function. When you use input()
function to get some numeric value from the user, you have to type-cast that data into an integer or a float, since the input() function always returns an string data-type. But, you don't have to convert the returned value from the input() function to string data-type, when concatenating to other strings.
day_night = "Midnight"
exact_time = 12
# exact_time = str( 12 ) # You can also type-cast before
# or you can ask the user (public) to enter age
# exact_time = input( "What is your birth time: " ) # type-casting is not needed
print( "My time of birth is " + day_night + " at " + str( exact_time ) + "." )
While concatenating symbols, such as full-stop, comma, plus sign, minus sign, etc. as string, you should be first assured about the positions as described in above example, that is, when adding full-stop, a blank (white-) space may be added after it, when there is next sentence. Any word or number which would follow some texts, a blank (white-) space should be provided before that word or number, and so on...
Type Casting Strings to Integers, and Concatenation
print( '123' + '654' ) # Outputs 123654
print( int( '123' ) + int( '654' ) ) # Outputs 777
Type Casting Integers to Strings, and Concatenation
print( 123 + 654 ) # Outputs 777
print( str( 123 ) + str( 654 ) ) # Outputs 123654
Using \ Escape Characters
When you need a single quote or a double quote to be placed in a string literal, you have to use \ (backslash character) before them to escape from closing the string. You can also use alternate pair of double/
# my_string = 'Don't rely on floats.' # SyntaxError: unterminated string literal will be shown because after Don, the string literal is closed.
my_string = 'Don\'t rely on floats.' # Correct way to insert a single quote.
print( my_string ) # Outputs Don't rely on floats.
# my_mood = "I am "happy" now." # SyntaxError: invalid syntax will be shown
my_mood = "I am \"happy\" now." # Correct way to insert one or more double quotes.
print( my_mood ) # Outputs I am "happy" now.
print( "Don't rely on floats." ) # Outputs Don't rely on floats.
print( 'I am "happy" now.' ) # Outputs I am "happy" now.
join()
You can get help documentation, by executing the print( help( str.join ) ) statement. See below table for details. The join()
method takes only one argument, therefore, two (or more) strings are provided as items/
The join()
and split()
methods (see previous page) are used to convert a list to string and vice-versa. These two methods are opposite of each other. It is important to note that syntax of join()
method is slightly different from that of split()
method.
# Syntax: separator.join( iterable_data ) # iterable_data means list, tuple, dictionary, etc. When comma or other symbol is used as separator, fancy result may be returned, which may not be useful.
# Syntax: string_data.split( separator ) # By default, a blank (white-) space, new line, carriage return, line-feed, are treated as separator. You can also use comma, or other symbol as separator.
first_word = 'Good'
last_word = 'Morning'
print( " ".join( [first_word, last_word] ) ) # concatenate two strings, outputs Good Morning
my_string = "Hello World"
new_list = my_string.split() # see previous page to split strings
print( new_list ) # Outputs ['Hello', 'World']
new_string = " ".join( new_list )
print( new_string ) # Outputs Hello World
String is a sequence data-type, hence, can be used as iterable
print( '*'.join( str( 123456 ) ) ) # Outputs 1*2*3*4*5*6
my_string = 'PYTHON'
new_list = '*'.join( my_string )
print( new_list ) # Outputs P*Y*T*H*O*N
modified_list = new_list.split( '*' )
print( modified_list ) # Outputs ['P', 'Y', 'T', 'H', 'O', 'N']
new_string = ''.join( modified_list ) # Here, empty string (without a blank space) is used as separator
print( new_string ) # Outputs PYTHON
replace()
As name of the method suggest, replace()
method is used to replace the old-string to new-string. However, you can also use some placeholder, which can be replaced with some value. Hence, in this way, you can concatenate some data (string or type-casted to string) into parent string. You can get help documentation, by executing the print( help( str.replace) ) statement.
parent_string = 'Hello World'
new_string = parent_string.replace( 'Hello', 'New' )
print( new_string ) # Outputs New World
my_string = 'Hello World'
modified_string = my_string.replace( 'l', 'm', 2 )
print( modified_string ) # Outputs Hemmo World because only first sub-
method/ | returned type | returned value |
---|---|---|
join(self, iterable, /) | str | Concatenate any number of strings. The string whose method is called is inserted in between each given string. |
replace(self, old, new, count=-1, /) | str | Return a copy with all occurrences of substring old replaced by new. If the optional argument count is given, only the first count occurrences are replaced. |
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
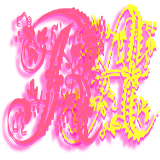
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇