
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Slicing Strings, Lists and Tuples in Category: Python by amit
🕙 Posted on 2023-08-05 at 07:10:14 Read in Hindi ...
List, Tuple, Dictionary & Slicing
You should remember that strings and tuples are immutable data-types, that is, they are not changeable. You have learned in previous pages, about various string methods, concatenation, etc. It means that any built-in function for example, len()
, any of string methods, concatenation applied on a string literal, or extraction of a single character (using []
square brackets just after the variable name, or string literal itself), doesn't change the original value.
If you want to use the result/print()
function only displays the result of evaluated expressions or statements within its parentheses.
Example 1
my_string = 'caMelcaSe stRing'
string1 = my_string.title()
string2 = my_string.capitalize()
string3 = my_string.upper()
string4 = my_string.lower()
print( my_string ) # Outputs the original string
There is no concept of CONSTANTS in Python, unlike other programming languages. And, thus, you can notice that a variable name can be assigned with a value (data) or re-assigned to another value, but those values may be of string or tuple data-type (literals), and thus immutable. Python is much memory efficient, because it stores a particular value (data) at only one memory location.
However, list and dictionary data-types are mutable (changeable), and much more flexible and therefore, lists and dictionaries are frequently used after strings in Python. You can see that the string data-type has much more methods than other data-types. The three data-types, strings, lists and tuples are indexed based sequence data-type. It means that like strings, each individual item/
Example 2
my_string = "Hello World"
my_list = [ "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" ]
my_tuple = ( "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" )
print( my_string, len( my_string ), type( my_string ) )
print( my_list, len( my_list ), type( my_list ) )
print( my_tuple, len( my_tuple ), type( my_tuple ) )
print( my_string[-1], my_list[-1], my_tuple[-1] ) # Outputs d d d
print( my_string[ -len( my_string ) ], my_list[ -len( my_string ) ], my_tuple[ -len( my_string ) ] ) # Outputs H H H
["H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d"] 11 <class 'list'>
("H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d") 11 <class 'tuple'>
d d d
H H H
In the above example, you can see that length of each of these three variables, my_string, my_list and my_tuple is 11. There is a blank space inside each of these variables, which is also counted as a character (of my_string) or an item/
You should also note that an empty string (without any blank space within a pair of single/
Example 3
my_string = ''
my_list = [ '' ]
my_tuple = ( '', ) # Remember: a single item/
my_dict = { 'empty': '' } # a dictionary must have key: value pair
my_set = { '' } # otherwise, it will be a set data-type
print( my_string, len( my_string ), type( my_string ) )
print( my_list, len( my_list ), type( my_list ) )
print( my_tuple, len( my_tuple ), type( my_tuple ) )
print( my_dict, len( my_dict ), type( my_dict ) )
print( my_set, len( my_set ), type( my_set ) )
[''] 1 <class 'list'>
('',) 1 <class 'tuple'>
{'empty': ''} 1 <class 'dict'>
{''} 1 <class 'set'>
In the above example, there is only one item/
However, to access an item/
Example 3 (continued)
# print( my_string[0] ) # there is no character in empty string literal
print( my_list[0], len( my_list[0] ), type( my_list[0] ) )
print( my_tuple[0], len( my_tuple[0] ), type( my_tuple[0] ) )
print( my_dict['empty'], len( my_dict['empty'] ), type( my_dict['empty'] ) )
# print( my_set[0], len( my_set[0] ) ) # Outputs TypeError: 'set' object is not subscriptable
0 <class 'str'>
0 <class 'str'>
Slicing of STRINGs, LISTs, and TUPLEs
Generally, slicing is done with two arguments (separated by colon symbol) within []
square brackets just after the variable name or string/
string_literal[start:stop:step]
list_literal[start:stop:step]
tuple_literal[start:stop:step]
start
is the starting index number (inclusive) of the string literal, list literal, or tuple literal. Similarly, stop
is the index number (exclusive) of that literal or variable name, before which slicing is done. It means that when you write [1:4], slicing will be done from index number 1 to 3. The third argument, step
is positions which will be iterated, that is, when step is 1 (by default), no character or item/
start
and stop
arguments take only integer data value (positive or negative index number), but step
argument takes only positive integer value. These three arguments are optional, and can be left blank before and after : (colon) symbol. When start is left blank, the index number is 0 (zero), and when stop is left blank, the slicing will be done upto end of string/
In the following table, you can see that each character (of String data-type), or each item/
H | e | l | l | o | W | o | r | l | d | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
-11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 |
Example 4
You can also use negative index numbers for start
and stop
arguments.
my_string = "Hello World"
my_list = [ "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" ]
my_tuple = ( "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" )
sliced_string = my_string[1:4]
sliced_list = my_list[1:4]
sliced_tuple = my_tuple[1:4]
print( sliced_string, type( sliced_string ) ) # Outputs ell <class 'str'>
print( sliced_list, type( sliced_list ) ) # Outputs ['e', 'l', 'l'] <class 'list'>
print( sliced_tuple, type( sliced_tuple ) ) # Outputs ('e', 'l', 'l') <class 'tuple'>
Example 5
When two or more characters / items in a string/step
is used. Therefore, numbers are used in example 5, to distinugish that second and fourth characters/
my_string = "123456789" # this is a string data-type
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9] # this is list of nine items
my_tuple = (1, 2, 3, 4, 5, 6, 7, 8, 9) # this is tuple of nine items
sliced_string = my_string[0:5:2]
sliced_list = my_list[0:5:2]
sliced_tuple = my_tuple[0:5:2]
print( sliced_string ) # Outputs 135
print( sliced_list ) # Outputs [1, 3, 5]
print( sliced_tuple ) # Outputs (1, 3, 5)
Example 6
In the following example 6, the slice()
built-in function is used with three arguments separated by , (comma) symbol to slice the string literal "Hello World", the list literal ["H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d"], and the tuple literal ("H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d"). In below example, first 'l' letter (index number 2), first 'o' letter (index number 4) and 'W' letter (index number 6) are skipped when slice( 1, 8, 2 )
is applied on each literal.
my_string = "Hello World"
my_list = [ "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" ]
my_tuple = ( "H", "e", "l", "l", "o", " ", "W", "o", "r", "l", "d" )
sliced_string = my_string[slice(1,8,2)]
sliced_list = my_list[slice(1,8,2)]
sliced_tuple = my_tuple[slice(1,8,2)]
print( sliced_string, type( sliced_string ) ) # Outputs el o <class 'str'>
print( sliced_list, type( sliced_list ) ) # Outputs ['e', 'l', ' ', 'o'] <class 'list'>
print( sliced_tuple, type( sliced_tuple ) ) # Outputs ('e', 'l', ' ', 'o') <class 'tuple'>
print( help( slice ) ) # Few lines of slice() help documentation is shown below:
Help on class slice in module builtins:
class slice(object)
| slice(stop)
| slice(start, stop[, step])
|
| Create a slice object. This is used for extended slicing (e.g. a[0:10:2]).
|
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
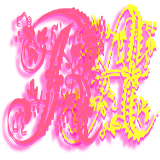
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇