
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Strings in Category: Python by amit
🕙 Posted on 2023-07-10 at 22:06:03 Read in Hindi ...
Sequence Data Types
Booleans and numbers (integers and floats) are primitive data-types. But strings, lists, tuple, dictionary, etc. are sequence data types, which are collection of either characters or elements (items). Let's see each of them in details. There is no char or character data-type in Python programming language, unlike C, C++, Java, etc. A single character within a pair of single quotes or a pair of double quotes is a string literal.
Strings
You have seen various strings in previous pages, for example, 'Hello', "Hello World", '12', Greeting = 'Hello World', name = 'Amit', etc. The first three are string literals on which print()
built-in function is applied, and the last two are string literals, both of which are assigned to variable names, namely Greeting and name.
Caution
: You should be careful when writing string literals, because even a single letter (character) without any pair of quotes, will be treated as variable name, (whether defined or undefined) and therefore, may cause BUGs. In the following example, the word Greeting is a variable name, but when it is enclosed within a pair of single quotes, it is treated as string data-type.
Greeting = 'Hello World'
print( Greeting ) # outputs Hello World
print( 'Greeting' ) # outputs Greeting
Different Ways of Writing Strings
String literals can be written in four ways, that is, one or more characters enclosed within (1) a pair of single quotes, (2) a pair of double quotes, (3) a pair of three single quotes, and (4) a pair of three double quotes. Last two ways are called docstrings, and multi-line characters, words, paragraphs, are written, especially documentation for your custom modules, functions, etc.
string1 = 'Hello World'
string2 = "Good Morning"
string3 = '''
# You should be careful when writing string literals,
# because even a single letter (character) without any
# pair of quotes, will be treated as variable name,
# (whether defined or undefined) and therefore, may
# cause BUGs.
''' # end of single three quotes docstring
string4 = """
# There are many built-in functions, and pre-defined
# methods for each of these functions in Python programming
# language. Many method names are same for different
# built-in functions.
""" # end of double three quotes docstring
print( string1, string2, string3, string4 )
In the above example, all four variable names, namely, string1, string2, string3, string4 are executed with one print()
function. Therefore, values of these four variable names, should be placed in one line. However, when you place characters, words, or paragraphs in new line, then these new lines are preserved, and therefore, output is as follows:
C:\xampp\
Hello World Good Morning
# You should be careful when writing string literals,
# because even a single letter (character) without any
# pair of quotes, will be treated as variable name,
# (whether defined or undefined) and therefore, may
# cause BUGs.
# There are many built-in functions, and pre-defined
# methods for each of these functions in Python programming
# language. Many method names are same for different
# built-in functions.
You can see that even in the output of above example, the # (hash) symbol doesn't comment any line, which are within a pair of three single/
However, docstrings are string literals, which when used as multi-line comments, that is, without assigning to any variable name, are temporarily stored in RAM of your local computer or remote server. When large amount of docstrings are used in your code, it may cause performace issues, such as slow loading of web-pages, hanging, etc.
Indexing of String Literals
A string literal is the collection of one or more characters. Each character, including blank space has some position, and it can be represented as index number of that character. You can access individual character from a string literal, with help of these index numbers. The first index number is (from left to right,) 0 (zero), and the last index number is len( 'H' ) - 1 that is, when there is only one character in a string literal, it is 0.
From left: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, ...
From right: -1, -2, -3, -4, -5, -6, -7, -8, -9, -10, -11 ...
The last index number of a string literal, can also be accessed with negative integers, that is -1 (from right to left). Thus, first index number is - len( 'H' ) (negative of len() output). The index number can only be integer values, and not floats, etc. The value of a character in a string literal can be accessed by mentioning index number with []
(square brackets), just after the string literal or variable name, to which it is assigned.
In the following example, you can see that first character from left, of variable name, my_string is accessed either by
my_string[0] # (positive) or
my_string[ -len( my_string ) ] # (negative).
# The second character from left, can be accessed either by
my_string[1] # (positive) or
my_string[ -len( my_string ) + 1 ] # (negative), and so on.
# Similarly, the last character from left, can be accessed either by
my_string[ ( len( my_string ) - 1 ) ] # (positive) or
my_string[ -1 ] # (negative).
# And, the second last character from left, can be accessed either by
my_string[ ( len( my_string ) - 2 ) ] # (positive) or
my_string[ -2 ] # (negative).
my_string = 'Hello World'
print( my_string[0] ) # character at first position from left, outputs H
print( my_string[1] ) # character at second position from left, outputs e
print( my_string[-1] ) # character at last position from left, outputs d
print( my_string[-2] ) # character at second position from right, outputs l
length_of_my_string = len
( my_string )
print( length_of_my_string ) # Outputs 11
print( my_string[ -length_of_my_string ] ) # character at last position from right, outputs H
print( my_string[ (-length_of_my_string + 1) ] ) # character at second last position from right, outputs e
print( my_string[ length_of_my_string - 1 ] ) # character at last position from left, outputs d
print( my_string[ length_of_my_string - 2 ] ) # character at second last position from left, outputs l
# subtracting from negative number will give more negative value
# for example, -5 - 1 = -6, but -5 + 1 = -4
If you are confused with above example, simply follow the below table. But, in real-world example, when length of a string is not known, you have to use len() built-in function which outputs total number of characters in a string. Since the positive index number starts with 0 (zero), the output of len() function is always one more than last index number. Also, the negative index number (from right to left) starts with -1 (minus one), therefore the last character from right to left (or first character from left to right) will be negative of output of len() function.
H | e | l | l | o | W | o | r | l | d | |
---|---|---|---|---|---|---|---|---|---|---|
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
-11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 |
my_string = 'Hello World'
# Notice that the blank space is displayed in new linefor
one_char in
my_string:
Generally, for
loop is used to extract the single character or single item from a sequence data-type. In next pages, you will learn about string methods, and other data-types.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
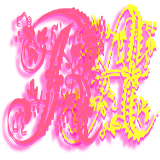
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇