
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Numbers with Decimal Points in Category: PHP by amit
🕙 Posted on 2023-05-04 at 00:45:37 Read in Hindi ...
Floats and Mathematical Operators
Float or double (also known as floating point numbers) are fractional (rational or irrational) decimal numbers. In previous page, you have learned that decimal numbers are of base 10, that is, these numbers are consisted of digits from 0
to 9
. Binary numbers are of base 2, Octal numbers are of base 8, and Hexadecimal numbers are of base 16.
Integers (which can be represented in all these bases), are stored in computer memory (RAM), in binary system. However, it is not possible to store float or fractional numbers in the same way. Therefore, computer engineers and developers had gone another way to store floats internally. And, thus, floating point numbers are of limited precision and you should not trust on floats to the last digits.
No matter how much powerful a Computer is, its CPU and RAM have limited power to calculate mathematical fractions, and there may be ERROR when you want to compare them, or do another operations. The size of a float is platform-dependent, although a maximum of approximately 1.8e308 with a precision of roughly 14 decimal digits is a common value (the 64 bit IEEE format).
<?php
echo PHP_FLOAT_MAX; // 1.7976931348623E+308
echo '<br />';
echo PHP_FLOAT_MIN; // 2.2250738585072E-308
(not negative number)
echo '<br />';
echo PHP_FLOAT_SIZE; // error, no such pre-defined constant exists?>
2.2250738585072E-308
Fatal error: Uncaught Error: Undefined constant "PHP_FLOAT_SIZE" in C:\xampp
Floating point numbers can be represented in following ways. e
or E
is the exponential power of 10 (that is, 7e1 is equal to 70, 7e2 is equal to 700, 7e-1 is equal to 0.7, and 7e-2 is equal to 0.07, and so on.) You must remember that negative number (integer or float) after e
or E
does not make the value negative, but it sub-divide exponentially the number (either integer or float) before it. Square root and cube root of a number can be achieved by exponentially sub-dividing by ½ (one-half) and ⅓ (one-third).
<?php
echo 12.3456789; // outputs 12.3456789
echo '<br />'; echo 12.3e3; // outputs 12300
echo '<br />'; echo 12.3e-3; // 0.0123
echo '<br />'; echo 123_456.789; // 123456.789 // as of PHP 7.4.0
echo '<br />'; var_dump( 7E-10 ); // float(7.0E-10)?>
Irrational Numbers
Irrational numbers are those which cannot be represented completely in digits. You have seen an example of PI in the movie ‘Life of Pi’ when the young boy had tried to write its value to the last digit! Other examples of irrational numbers are square root of 2, 3, 5, etc. (that is, square root of prime numbers). To explain how irrational numbers are written in programming languages, you should first know about operators used in these (mathematical) operations.
Operators
Arithematic Operators
There are different types of operators, for example, arithematic operators, assignment operators, logical operators, comparison operators, bitwise operators, etc. Unlike common mathematical operators tought in school, arithematic operators in PHP (and other programming languages, such as Python, JavaScript, C, C++, Java) are + (addition), - (subtraction), * (multiplication), / (division), % (modulus or remainder), ** (exponential), etc.
Assignment Operators
Assignment operators are used to assign (place) value to a variable or a constant (using const
keyword). These are = (simple assignment), += (addition assignment), -= (subtraction assignment), *= (multiplication assignment), /= (division assignment), %= (modulo assignment), **= (exponential assignment), etc. Do not confuse = as equal to operator, which is explained below.
Comparison Operators
Numbers, string, array, boolean values, etc. can be compared to another number, string, array, etc. (it is not necessary that same data-type should be compared!) Comparison always returns a boolean value, that is, 'true' or 'false'. These are == and != ('equal to' and 'not equal to' - loose comparison, only checks the comparison of values), === and !== ('identical to' and 'not identical to' - strict comparison, checks the comparison of values and also data-type).
Other comparison operators are < (less than), > (greater than), <= (less than or equal to), >= (greater than or equal to), <> (less than or greater than, that is, not equal), <=> (it is known as spaceship operator, returns -1
when less than, 0
when equal to, and 1
when greater than). Prior to PHP 8.0.0, when a string (not number enclosed in quotes) is compared with another number or numeric string, comparison is done numerically. This can lead to surprising results. You will learn more about these operators in next pages, as we move to advance sections of PHP.
Back to Irrational Numbers
Following irrational numbers can be obtained by sub-dividing **(1/2) that is, half-exponential of integers. You can also try to obtain cube-root of 2, 3, etc. using same method, for example, 2**(1/3), 3**(1/3) and so on. These irrational numbers are not precise and limited to some extent. When you use echo
function to get value from following example, instead of var_dump()
, the results will be less precise.
<?php
var_dump( 22/7 ); // value of PI
echo '<br />'; var_dump( 2**0.5 ); // square root of 2
echo '<br />'; var_dump( 3**0.5 ); // square root of 3?>
float(1.4142135623730951)
float(1.7320508075688772)
Never Trust Floating Point Numbers
You have seen in the above examples that floats are of limited precision. Therefore, you should never compare floating point numbers, and should not believe on mathematical operations even on rational numbers. 0.5, 2.3435642564 or any floating point number, which is an output from PHP (or other programming languages) are rational numbers, because they have been represented after decimal point (dot) completely.
<?php
echo (int)
( (0.1+0.7) * 10 ); // outputs 7
echo '<br />'; var_dump( (0.1+0.7) * 10 ); // float(7.999?>
In the above code, you have seen that type-casting floating point numbers to integers will remove all digits after the decimal point (dot). Thus, the value is always truncated, and the result will be either equal to or less than original floating point number. In next pages, you will learn more about type-casting of different data-types in other data-types, including floats. Also, you will learn about Math functions which are also applied on floating point numbers. Describing everything in this page will overwhelm you, and also the topic will become too long!
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
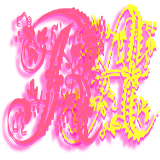
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇