
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
More Operators in Category: PHP by amit
🕙 Posted on 2023-06-02 at 21:12:26 Read in Hindi ...
More about Operators
There are many more different kinds of operators in PHP. You have seen in previous page about Conditional Control Flow Structures. In if(){} elseif(){} else{}
conditional blocks, only series of statements inside one block, i.e. {}
curly braces, will be executed, and other blocks are discarded. Similar effects are also achieved by some operators, for example −
condition :
statement1 ?
statement2 (ternary operator)
<?php
$my_var = false; // variable $my_var is used as condition
$result_var = $my_var ?
"Correct" :
"Incorrect"; // result of ternary operator is always assigned to a variable
echo $result_var; // Outputs Incorrect
echo '<br />';?>
In the above example, the ternary operator requires three operands − first is conditional expression, second is a statement1, which will only be executed when the conditional expression is true
, and third is statement2, which will be executed only when the conditional expression is false
. The above example is same as following if(){} else{}
block.
<?php if( false ) echo "Correct"; else echo "Incorrect"; ?>
You can see that output from a ternary operator expression is assigned to a variable name, but the if(){} else{}
block executes the code directly. Both way of writing codes are important, and will be used in scripts wherever necessary. You should use parentheses ()
when stacking ternary operators. Consider following examples −
<?php echo true ? 'true' : false ? 't' : 'f'; // Output is shown below ('t' prior to PHP 7.4). ?>
Fatal error: Unparenthesized `a ? b : c ? d : e` is not supported. Use either `(a ? b : c) ? d : e` or `a ? b : (c ? d : e)` in C:\xampp\
<?php echo true ? 'true' : (false ? 't' : 'f'); // outputs true when used in parenthesis ?>
<?php echo (true ? 'true' : false) ? 't' : 'f'; // outputs 't' ?>
<?php echo false ? 'true' : (false ? 't' : 'f'); // outputs 'f' ?>
It is recommended to avoid "stacking" ternary expressions. PHP's behaviour when using more than one unparenthesized ternary operator within a single expression is non-obvious compared to other languages. Indeed prior to PHP 8.0.0, ternary expressions were evaluated left-associative, instead of right-associative like most other programming languages. Relying on left-associativity is deprecated as of PHP 7.4.0. As of PHP 8.0.0, the ternary operator is non-associative.
expression1 ??
expression2 (null coalescing).
Do not use ??
null coalescing operator, unless necessary. This operator is applied on two operands − most operators in PHP and other programming languages are binary. You should not misunderstood binary operators with binary numbers. = (assignment operator) requires two operands − the right operand or expression is evaluated and assigned to variable/?:
as explained above.
Unary operator means there is only one operand, on which it is applied − for example +
(prefix) and -
(prefix) are identity and negation (different than addition and subtraction, which are binary operators); ++
(increment) and --
(decrement) operators; !
(not) and ~
(tilda key left of 1 in your keyboard, use Shift key). Different kinds of operators are explained in previous page.
<?php $abc = null; $xyz = $abc ?? "hello"; echo $xyz; // Outputs hello ?>
<?php $abc = false; $xyz = $abc ?? "hello"; var_dump( $xyz ); // Outputs bool(false) ?>
<?php $xyz = $mno ?? "hello"; echo $xyz; // Outputs hello ?>
In above three examples, you can see that ??
(null coalescing) operator outputs the second operand, when the first operand is null
or not defined (undefined), otherwise it outputs the first operand. In this way you can assign a default value to the result output when any variable/null
. You can also use isset()
function, as explained in previous page.
Bitwise Operators ~ & | ^ << >>
These bitwise operators (except ~) are binary operators, that is, each of these require two operands. ~
(tilda) operator is unary operator. The PHP interpreter first converts the operands to binary representation, and then the bitwise operations are done on them. You can directly get the output with echo
or var_dump()
function, or assign them to a variable/
Operator | Operation | Binary Representation | Binary Result | Output |
---|---|---|---|---|
& (bitwise and) | 6 & 3 | 110 & 011 | 010 | 2 |
| (bitwise or) | 6 | 3 | 110 | 011 | 111 | 7 |
^ (bitwise xor) | 6 ^ 3 | 110 ^ 011 | 101 | 5 |
^ | 5 ^ 2 | 101 ^ 10 | 111 | 7 |
^ | 5 ^ 4 | 101 ^ 100 | 001 | 1 |
~ (bitwise not) | ~ 6 | ~110 (flipped to →) | 001 | -7 |
~ | ~6 & 3 | 001 & 011 | 001 | 1 |
<< (bitwise shift left) | 6 << 3 | 110 is shifted to left by 3 zeros | 110000 | 48 |
>> (bitwise shift right) | 6 >> 3 | 110 is shifted to right by 3 zeros | 000 | 7 |
>> | 6 >> 1 | 110 is shifted to right by 1 zero | 011 | 3 |
&
(bitwise and) compares respective positions in two operands, and when both are 1, then outputs 1, otherwise 0 (zero) is output.
<?php var_dump( 6 & 3 ); // outputs int(2) ?>
|
(bitwise or) compares respective positions in two operands, and when both are 0, then outputs 0, otherwise 1 (one) is output.
<?php var_dump( 6 | 3 ); // outputs int(7) ?>
^
(bitwise xor) compares respective positions in two operands, and when both are opposite of each other, then outputs 1, otherwise 0 (zero) is output. decbin()
(decimal to binary) pre-defined Math function and (HTML whitespace) or <br /> (HTML line-break) can be used to describe the output as shown below −
<?php var_dump( 6 ^ 3 ); // outputs int(5) ?>
<?php echo decbin(5), ' ', decbin(2), ' ', decbin(5^2), ' ', 5^2; // outputs 101 10 111 7 ?>
<?php echo decbin(5), ' ', decbin(4), ' ', decbin(5^4), ' ', 5^4; // outputs 101 100 1 1 ?>
~
(bitwise not) compares respective positions in the single operand, and flipps it to opposite. The binary result of opposite will become the output.
<?php var_dump( ~ 6 ); // outputs int(-7) ?>
<?php var_dump( ~ 6 & 3 ); // outputs int(1) ?>
<<
(bitwise shift left) and >>
(bitwise shift right) shift the binary digits by 0
(zeros) to left and right repectively, the binary result will be converted to decimal (integer). Binary digits are 0 and 1, whereas decimal digits are from 0 to 9.
<?php var_dump( 6 << 3 ); // outputs int(48) ?>
<?php var_dump( 6 >> 3 ); // outputs int(0) ?>
<?php var_dump( 6 >> 1 ); // outputs int(3) ?>
Logical Operators ! && || and or xor
Logical operators are applied on boolean valuation of any literal operands, and they always outputs boolean results. These logical operators are frequently used in control structures to evaluate complex conditional expressions. Let't explain each of these logical operators, one-by-one −
&&
(logical and) has higher operator precence over and
(reserved keyword), but both behaves as same. When both operands are evaluted as true
, then the output is true, otherwise output will be false.
<?php var_dump( 1 && 1 ); // outputs bool(true) ?>
<?php var_dump( 1 && 0 ); // outputs bool(false) ?>
<?php var_dump( 0 && 0 ); // outputs bool(false) ?>
||
(logical or) has higher operator precence over or
(reserved keyword), but both behaves as same. When any one of two operands are evaluted as true
, then the output is true, otherwise output will be false.
<?php var_dump( 1 || 1 ); // outputs bool(true) ?>
<?php var_dump( 1 || 0 ); // outputs bool(true) ?>
<?php var_dump( 0 || 0 ); // outputs bool(false) ?>
xor
(logical xor) outputs true when either of two operands is evaluated as true
, but not both. That is when both operands are evaluated as same boolean value, the output is false.
<?php var_dump( 1 xor 1 ); // outputs bool(false) ?>
<?php var_dump( 1 xor 0 ); // outputs bool(true) ?>
<?php var_dump( 0 xor 0 ); // outputs bool(false) ?>
!
(logical not) outputs the opposite of boolean valuation of a literal or variable/
<?php var_dump( ! 0 ); // outputs bool(true) ?>
<?php var_dump( ! 1 ); // outputs bool(false) ?>
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
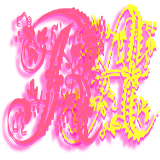
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇