
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Type Casting in Details in Category: PHP by amit
🕙 Posted on 2023-05-22 at 20:22:55 Read in Hindi ...
Type Casting
You have seen some type-casting in previous pages, but in this topic, it will be described elaborately. As a programmer, you should think on every possible options in which a user can input data. Since PHP is a loose data-type scripting language, you should be carefully examine every data, you may encounter.
Boolean to Other Data-types
When true is type-casted to integer
or float
data-type, it is converted to 1
. When false is type-casted to integer
or float
data-type, it is converted to 0
.
<?php var_dump( (int) true
); // int(1) ?><br />
<?php var_dump( (int) false
); // int(0) ?><br />
<?php var_dump( (float) true
); // float(1) ?><br />
<?php var_dump( (float) false
); // float(0) ?><br />
When true and false are type-casted to (string)
data-type, they are converted to "1" and "" (empty string) respectively.
<?php var_dump( (string) true
); // string(1) "1" ?><br />
<?php var_dump( (string) false
); // string(0) "" ?><br />
When true and false are type-casted to (array)
data-type, they are added as first item (element) to an empty array. The values of those items are respectively same as those boolean values.
<?php var_dump( (array) true
); // array(1) { [0] => bool(true) } ?><br />
<?php var_dump( (array) false
); // array(1) { [0] => bool(false) } ?><br />
Integer to Other Data-types
An integer can be negative, zero, or positive number. Outputs from following codes are shown in comment, which is self-explanatory. When 0
is type-casted to (boolean)
data-type, the output is false. All other values are treated as true.
<?php var_dump( (bool) -5 ); // bool(true) ?><br />
<?php var_dump( (bool) 0 ); // bool(false) ?><br />
<?php var_dump( (bool) -5 ); // bool(true) ?><br />
When any integer is type-casted to float or string data-type, its value is same, as shown below in comments. When 0
is type-casted to (string)
, it is converted into an empty string.
<?php var_dump( (float) -3 ); // float(-3) ?><br />
<?php var_dump( (float) 0 ); // float(0) ?><br />
<?php var_dump( (float) 3 ); // float(3) ?><br />
<?php var_dump( (string) -4 ); // string(2) "-4" ?><br />
<?php var_dump( (string) 0 ); // string(0) "" ?><br />
<?php var_dump( (string) +4 ); // string(1) "4" ?><br />
When any integer value is type-casted to (array)
data-type, it is added as first item of an empty array. Output is shown in comments.
<?php var_dump( (array) -6 ); // array(1) { [0] => int(-6) } ?><br />
<?php var_dump( (array) 0 ); // array(1) { [0] => int(0) } ?><br />
<?php var_dump( (array) +6 ); // array(1) { [0] => int(6) } ?><br />
Floating Point Numbers to Other Data-types
Other than negative, zero, and positive floating point numbers, there are reserved keywords, INF
, -INF
, NAN
,M_PI
, etc. which are also float data-type. Type-casting of these (pre-defined) constants are as follows:
<?php var_dump( (bool) INF ); // bool(true) ?><br />
<?php var_dump( (bool) -INF ); // bool(true) ?><br />
<?php var_dump( (bool) NAN ); // bool(true) ?><br />
<?php var_dump( (bool) M_PI ); // bool(true) ?><br />
In above codes, you can see that NAN (Not A Number) is not equal to bool(false). However, when these pre-defined constants, that is, INF, -INF and NAN are type-casted to (int)
or (integer)
, their values are all 0
. Constant M_PI is the value of pi()
function, and when type-casted to (integer)
, it is truncated to 3.
<?php var_dump( (integer) INF ); // int(0) ?><br />
<?php var_dump( (integer) -INF ); // int(0) ?><br />
<?php var_dump( (integer) NAN ); // int(0) ?><br />
<?php var_dump( (integer) M_PI ); // int(3) ?><br />
<?php var_dump( (string) INF ); // string(3) "INF" ?><br />
<?php var_dump( (string) -INF ); // string(4) "-INF" ?><br />
<?php var_dump( (string) NAN ); // string(3) "NAN" ?><br />
<?php var_dump( (string) M_PI ); // string(15) "3.1415926535898" ?><br />
<?php var_dump( (array) INF ); // array(1) { [0] => float(INF) } ?><br />
<?php var_dump( (array) -INF ); // array(1) { [0] => float(-INF) } ?><br />
<?php var_dump( (array) NAN ); // array(1) { [0] => float(NAN) } ?><br />
<?php var_dump( (array) M_PI ); // array(1) { [0] => float(3.141592653589793) } ?><br />
You can see in above codes that when these pre-defined constants are type-casted to (array)
data-type, these floating point numbers are added as first item to an empty array.
All floating point numbers (except pre-defined constants), that is, negative, zero and positive floating point numbers behave just like integers, except, when they are type-casted to (integer)
, the fractional part (digits after the decimal point/
Strings to Other Data-types
Strings can be classified as different values, for example, ""
(empty string, without any blank space within it), "+12.4"
(numeric string, either signed or unsigned, without underscore symbols), and "false"
(non-numeric string, whether characters within a pair of single/double quotes are pre-defined constants).
<?php var_dump( (bool) "" ); // bool(false) ?><br />
<?php var_dump( (bool) "-1.2" ); // bool(true) ?><br />
<?php var_dump( (bool) "false" ); // bool(true) ?><br />
<?php var_dump( (int) "" ); // int(0) ?><br />
<?php var_dump( (int) "-12.5" ); // int(-12) ?><br />
<?php var_dump( (int) "INF" ); // int(0) ?><br />
<?php var_dump( (float) "" ); // float(0) ?><br />
<?php var_dump( (float) "-12.5" ); // float(-12.5) ?><br />
<?php var_dump( (float) "INF" ); // float(0) ?><br />
<?php var_dump( (array) "" ); // Array (1) { [0] => string(0) "" } ?><br />
<?php var_dump( (array) "-12.5" ); // Array (1) { [0] => string(5) "-12.5" } ?><br />
<?php var_dump( (array) "INF" ); // Array (1) { [0] => string(3) "INF" } ?><br />
You can visit previous page to see more type-casting from string data-type to other data-type, and vice-versa.
Arrays to Other Data-types
Arrays can be classified into either empty array or non-empty array for purpose of type-casting to other data-types. Arrays cannot be type-casted to (string)
data-type. Conversion of an array to a string can be done with implode() function. Similarly, a string (with some separator) can be converted into an array, with explode() function.
<?php var_dump( (bool) []
); // bool(false) ?><br />
<?php var_dump( (int) []
); // int(0) ?><br />
<?php var_dump( (float) []
); // float(0) ?><br />
<?php var_dump( (string) []
); // shows ERROR as shown below ?><br />
Warning: Array to string conversion in C:\xampp\
string(5) "Array"
<?php var_dump( (bool) [ false ]
); // bool(true) ?><br />
<?php var_dump( (int) [ 0 ]
); // int(1) ?><br />
<?php var_dump( (float) [ 1.2 ]
); // float(1) ?><br />
<?php var_dump( (string) [ "" ]
); // shows ERROR as shown below ?><br />
Warning: Array to string conversion in C:\xampp\
string(5) "Array"
<?php var_dump( (bool)
[ false, true, null ] ); // no matter how many items are inside an array, output is bool(true) ?><br />
<?php var_dump( (int)
[ 23, 34, true, 6534 ] ); //no matter how many items are inside an array, output is int(1) ?><br />
<?php var_dump( (float)
[ 1.2, false, 234, 34.32 ] ); // no matter how many items are inside an array, output is float(1) ?><br />
NULL to Other Data-types
null
can be type-casted to other data-types. However, conversion of other data-types to null
is DEPRECATED as of PHP 7.2.0, and REMOVED as of PHP 8.0.0. Relying on this feature is highly discouraged. Casting a variable to null using (unset)
is also DEPRECATED as of PHP 7.2.0, and REMOVED as of PHP 8.0.0.
<?php var_dump( (bool) null
); // bool(false) ?><br />
<?php var_dump( (int) null
); // int(0) ?><br />
<?php var_dump( (float) null
); // float(0) ?><br />
<?php var_dump( (string) null
); // string(0) "" ?><!-- Output is empty string --><br />
<?php var_dump( (array) null
); // array(0) { } ?><!-- Output is empty array --><br />
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
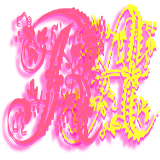
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇