
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Array Functions in Category: PHP by amit
🕙 Posted on 2023-05-20 at 16:33:56 Read in Hindi ...
Array Functions
array_values()
outputs the values of an array as items of an indexed array.
<?php $assoc_var = array
( 'xyz' => 1, 'abc' => 45, 'mno' => true ); ?>
<?php var_dump( array_values
( $assoc_var ) ); ?><br />
As you can see the below OUTPUT of above code, keys 'xyz', 'abc' and 'mno' are changed to 0, 1, and 2 respectively.
array_keys()
function finds all keys and returns them as items of an indexed array.
<?php var_dump( array_keys
( $assoc_var ) ); // variable $assoc_var is declared above ?><br />
When an item (or element) is associated with many keys, that is, an item (evaluated as same value) is placed in various place inside an array, array_keys()
function will return those keys, which are associated with that value (placed as second argument as shown below).
<?php var_dump( array_keys
( $assoc_var, 1 ) ); ?><br />
array_key_exists()
finds whethere a key exists or not in an array.
<?php var_dump( array_key_exists
("xyz", $assoc_var) ); // outputs bool(true) ?><br />
<?php var_dump( array_key_exists
(0, $assoc_var) ); // outputs bool(false) ?><br />
In the above example, the key 0
does not exist in an associative array $assoc_var and therefore, the output is bool(false).
Difference between array_key_exists()
and isset()
functions
isset()
function is generally used to check whether the value of a variable name is defined or not. In PHP, there is no undefined data-type, unlike JavaScript. When value of a variable name or an array key is null, isset()
function will output bool(false). Consider the following example (you can place numbers and a blank space after <br /> HTML element, to distinguish and recognize each output from others, as shown below):
<?php
$my_var = null;
echo '<br />1) '; var_dump( isset
( $my_var ) ); // $my_var is declared but, output is bool(false)
echo '<br />2) '; var_dump( isset
( $not_var ) ); // $not_var is neither declared nor assigned to any value. Output is bool(false)
$new_var = array
( 0, 1, null, '', false, null => 5, '' => 6 );
echo '<br />Is array set: ';
var_dump( isset
( $new_var ) ); // Output is bool(true)
echo '<br /> array is: ';
var_dump( $new_var ); // this array is mixture of indexed and associative keys
echo '<br /> 3) '; var_dump( isset
( $new_var[2] ) ); // Output is bool(false)
echo '<br /> 4) '; var_dump( isset
( $new_var[3] ) ); // Output is bool(true)
echo '<br /> 5) '; var_dump( isset
( $new_var[4] ) ); // Output is bool(true)
echo '<br /> 6) '; var_dump( isset
( $new_var[5] ) ); // $new_var[5] is not declared. Output is bool(false)?>
In above example, $my_var is assigned to null
, but output is bool(false). $not_var is not defined, and output is also bool(false). The last key of array $new_var that is ('' => 6) has replaced the previous key of that array, that is, (null => 5). ''
(empty string, a pair of single/false
as value of $new_var[3] and $new_var[4] respectively, are not null
, hence, output in both cases, are bool(true). There is no key $new_var[5] in above example, and echo
function will output ERROR along with NULL. Therefore, var_dump
( isset
( $new_var[5] ) ) outputs bool(false) as shown below.
1) bool(false)
2) bool(false)
Is array set: bool(true)
array is: array(6) { [0]=> int(0) [1]=> int(1) [2]=> NULL [3]=> string(0) "" [4]=> bool(false) [""]=> int(6) }
3) bool(false)
4) bool(true)
5) bool(true)
6) bool(false)
<?php echo $new_var[5]; ?>
Warning: Undefined array key 5 in C:\xampp\
min()
and max()
are Math functions, which are used to find minimum and maximum number in an array.
To find minimum: <?php echo min
( $assoc_var ); ?><br />
To find maximum: <?php echo max
( $assoc_var ); ?><br />
implode() and explode() functions are generally used to export/ import DATA from .csv files
.csv files are text files, in which DATA from spreadsheets, for example, MS-excel, etc. are stored with a separator, such as , (comma) or * (asterik) symbol. Many persons, who don't know about DATABASE or spreadsheet applications, are asked to save their data in simple .csv files. And, programmers converts those .csv files into other formats, as per their needs.
implode()
function converts an array into a string data-type
<?php $array_to_string = implode
( " * ", $assoc_var ); echo $array_to_string; ?><br />
explode()
function converts a string with some separation into an array data-type.
<?php $string_to_array = explode
( " * ", $array_to_string ); var_dump( $string_to_array ); ?><br />
In the above two examples, you can see that ' * ' (a blank space before and after the * symbol) is used as separator. A separator must be some unique character or characters, which is not generally used inside the DATABASE or .csv files as texts. You should be careful, while using a character as a separator.
For example, when you use only * symbol (without blank space) in either of above two functions, then you have to use only * (exactly same character) in the counterpart (opposite) function. It is also important to note that when you convert a string to array data-type, the output must not be displayed with echo
function, otherwise ERROR will be displayed in the web-browser!
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
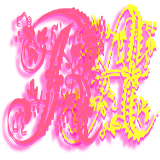
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇