
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
More Array Functions in Category: PHP by amit
🕙 Posted on 2023-05-21 at 14:33:11 Read in Hindi ...
More Array Functions
array_chunk()
splits an array into two or more arrays, and returns a NESTED array
array_chunk(array $array, int $length, bool $preserve_keys = false): array
The above line is the syntax of array_chunk() function. It means that within parentheses, the first argument is of array data-type, with variable name $array (or can be array literal). The second argument is of integer data-type, and variable name is $length (which is desired length of result arrays). The third argument is of boolean data-type, and variable name is $preserve_keys (it can only be true or false, false is default value).
The last word 'array' after : (semi-colon symbol) is the data-type of output (which is of array data-type). The third argument is optional, and you can be found syntaxes of all functions, as described in above line, in the PHP official documentation. When length of source array is not perfectly divisible by second argument passed in array_chunk() function, the last NESTED array within OUTPUT array will be of remainder (modulus) length.
<?php $numbers = [ 'abc' => 43, 'def' => 21, 'ghi' => 63, 'jkl' => 10, 'mno' => 87, 'pqr' => 4, 'stu' => 1, 'vwx' => 92 ]; ?>
<?php print_r( array_chunk
( $numbers, 3 ) ); // returns an indexed NESTED array ?>
<?php print_r( array_chunk
( $numbers, 3, true ) ); // returns an associative NESTED array ?>
You can think following functions as bank-balance (array), from which you withdraw or deposit amounts (items/
array_shift()
returns the first item from an array, after deducting that item from the same.
<?php
$my_var = array
( 23, 'hello', 9.23, true, 74, false, 92 );
var_dump( $my_var ); // before applying array_shift() function
$new_var = array_shift
( $my_var ); echo '<br />';
var_dump( $my_var ); // after applying array_shift() function
echo '<br />'; var_dump( $new_var );?>
array(6) { [0]=> string(5) "hello" [1]=> float(9.23) [2]=> bool(true) [3]=> int(74) [4]=> bool(false) [5]=> int(92) }
int(23)
array_pop()
returns the last item from an array, after deducting that item from the same.
<?php
$my_var = array
( 23, 'hello', 9.23, true, 74, false, 92 );
var_dump( $my_var ); // before applying array_pop() function
$new_var = array_pop
( $my_var ); echo '<br />';
var_dump( $my_var ); // after applying array_pop() function
echo '<br />'; var_dump( $new_var );?>
array(6) { [0]=> int(23) [1]=> string(5) "hello" [2]=> float(9.23) [3]=> bool(true) [4]=> int(74) [5]=> bool(false) }
int(92)
array_unshift()
prepends one or more items to an array, before all other items.
<?php
$my_var = array
( 'one' => 100, 'two' => 200, 'three' => 300 ); // array can be empty, without any item.
var_dump( $my_var ); // before applying array_unshift() function
array_unshift
( $my_var, 'hello', true, 23.54 );
echo '<br />';
var_dump( $my_var ); // after applying array_unshift() function?>
array(6) { [0]=> string(5) "hello" [1]=> bool(true) [2]=> float(23.54) ["one"]=> int(100) ["two"]=> int(200) ["three"]=> int(300) }
array_push()
appends one or more items to an array, after all other items.
<?php
$my_var = array
( 'one' => 100, 'two' => 200, 'three' => 300 ); // array can be empty, without any item.
var_dump( $my_var ); // before applying array_push() function
array_push
( $my_var, 'hello', true, 23.54 );
echo '<br />';
var_dump( $my_var ); // after applying array_push() function?>
array(6) { ["one"]=> int(100) ["two"]=> int(200) ["three"]=> int(300) [0]=> string(5) "hello" [1]=> bool(true) [2]=> float(23.54) }
Following array sorting functions are in couple, and are DESTRUCTIVE in nature:
That is one array function sorts from lower number to higher number or alphabets, and other sorts in reverse order. Also, no newer variable is needed for their returned output. The variable name to which array literal is assigned, will automatically be changed after operation with following functions.
<?php $numbers = [ 'abc' => 43, 'def' => 21, 'ghi' => 63, 'jkl' => 10, 'mno' => 87, 'pqr' => 4, 'stu' => 1, 'vwx' => 92 ]; ?>
sort(), rsort() − sort by value of items/ elements
<?php sort
( $numbers ); print_r( $numbers ); ?><br />
<?php rsort
( $numbers ); print_r( $numbers ); ?><br />
ksort(), krsort() − sort by key
CAUTION
: If you apply ksort(), krsort(), asort() and arsort() on the results gotten from either of the above two functions, that is, sort() or rsort(), the OUTPUT will be different. Therefore, apply each of these functions on original variable, after deleting previous functions in your .php file.
<?php $keys = [ 'xyz' => 43, 'mno' => 21, 'ghi' => 63, 'abc' => 10, 'stu' => 87, 'def' => 4, 'pqr' => 1, 'ghi' => 92 ]; ?>
<?php ksort
( $keys ); print_r( $keys ); ?><br />
<?php krsort
( $keys ); print_r( $keys ); ?><br />
asort(), arsort() − maintain index association − sorts in ascending/ descending order
<?php asort
( $keys ); print_r( $keys ); ?><br />
<?php arsort
( $keys ); print_r( $keys ); ?><br />
Most of array functions are rarely used in CMS (Content Management System), and these array functions will be explained later, whenever necessary. The syntax of each function in PHP official documentation is described as shown above.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
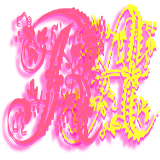
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇