
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Dealing with Individual Items of an Array in Category: PHP by amit
🕙 Posted on 2023-05-17 at 05:59:30 Read in Hindi ...
Manipulating Items of an Array
Remember the unset() (built-in/pre-defined) function, which not only destroys the value assigned to a variable name or a constant name, but it also destroys the variable name or the constant name. It means, that variable or constant has never existed in the REQUEST-RESPONSE CYCLE. Let't apply the unset()
function to an array.
<?php
$my_var = array
( "hero", 'zero', true
, 1, 2.5 );
unset
( $my_var[0] ); // destroys only the first key of the array
var_dump( $my_var ); // OUTPUT doesn't show the first key, 0
var_dump( $my_var[0] ); // outputs ERROR as the key 0 doesn't exist
unset
( $my_var ); // destroys the entire array
var_dump( $my_var ); // outputs ERROR as entire array doesn't exist
var_dump( $my_var[1] ); // you cannot access value from any key of an array, which doesn't exist?>
In above lines of code, unset( $my_var[0] ) will destroy only the first key, or unset( $my_var[1] ) will destroy only the second key, and so on... But unset( $my_var ) will destroy the entire array. In the following output, you can see that key [0] is missing from output of fourth line of code. Lines 5, 7 and 8 of above code, shows following outputs (NULL
), as key 0 or variable name $my_var is undefined. Line 8 outputs two warnings: (1) undefined variable name, and (2) trying to access key from NULL.
Warning: Undefined array key 0 in C:\xampp\
NULL
Warning: Undefined variable $my_var in C:\xampp\
NULL
Warning: Undefined variable $my_var in C:\xampp\
Warning: Trying to access array offset on value of type null in C:\xampp\
NULL
Though the above example is to explain that you should not oftenly use the unset()
function, to destroy a variable name or a constant name. Some of you may argue that variable or constant is already defined, and results can be obtained whenever someone refresh the web-browser. Remember that PHP is a scripting language, which outputs the result for one REQUEST-RESPONSE CYCLE. This means that no matter how many times you refresh this website, or any other web-page, a new REQUEST-RESPONSE CYCLE starts.
Therefore, it is necessary to replace the value of a variable or constant name without destroying itself, or generally, to replace the value of an item inside an array with NULL
or any other value, to avoid ERRORS as mentioned above. Let't see how this can be done, with the same example (without unset() function):
<?php $my_var = array
( "hero", 'zero', true
, 1, 2.5 ); ?>
<?php echo
$my_var[0]; // to access an item from the array ?><br />
<?php $my_var[0] = NULL
; // to change the value of an item ?>
<?php $my_var[3] = false
; // to change the value of an item ?>
<?php var_dump( $my_var ); ?><br />
array(5) { [0]=> NULL [1]=> string(4) "zero" [2]=> bool(true) [3]=> bool(false) [4]=> float(2.5) }
In the previous page also, you have seen that how value from an individual item of an array can be accessed (see above example). Similarly, you can use the index number (integer) or index key of other data-type, within the (opening and closing) square brackets just after the variable name or constant name of array data-type to add or to replace data into that array. Literal value of any data-type, supported by the PHP, or even a variable or constant can be assigned to an individual item of an array. In this way, you can also create a NESTED array.
Adding Item(s) in an Array
PHP is a loose data-type scripting language. This means that a variable name (or a constant name in one REQUEST-RESPONSE CYCLE) can hold any type of data, and at any time, the data-type of a variable name can be changed to another data-type. You can compel users to input data of specific data-type, but when these data are used inside the CMS (Content Management System) program, they can be type-casted into another data-types, or changed to another value.
It is very simple to add value of any data-type into an array, using (opening and closing) square brackets just after the variable name. It is also simple to add specific type of keys, for example, string keys, boolean keys, etc. into an associative array. In PHP, there is no difference between indexed array and associative array, except accessing or manipulating values through their keys. Let's create an empty array. Following examples will explain you everything I mentioned hereinabove.
<?php $new_var = array
(); // an empty array literal is assigned to variable, $new_var ?>
<?php $new_var[] = 'hello'; var_dump( $new_var ); ?><br />
<?php
$new_var = []
; /* empty array literal is re-assigned to variable, $new_var
It is not necessary to declare a variable name, and assign empty array literal to it. But, when same variable name is already declared, you can re-assign any value of any data-type. */
$new_var = true
; echo $new_var; // Now, it is not an array data-type.
echo '<br />';
$new_var = array()
; /* important: The above line makes the variable $new_var of scalar data-type, and when you don't write this line, or comment this line, ERROR will be shown as below */
$new_var[] = 3.14; print_r( $new_var ); echo '<br />';
$new_var[] = 'Amit'; print_r( $new_var );?>
<br />
Fatal error: Uncaught Error: Cannot use a scalar value as an array in C:\xampp\
Array ( [0] => 3.14 [1] => Amit )
From the above two examples, it is clear to you that (opening and closing) square brackets, without any key inside them, that is []
will add a new item to an array. These square brackets just after the variable name, will not replace the previous value to newer value. The second line in above OUTPUT shows that the variable name, $new_var now consists of two items. In next page, you will see various pre-defined functions, specifically used for arrays, to insert items at the beginning or at the last of already constituted keys or items into it.
<?php $new_var[] = $new_var; /* without re-assigning empty array literal, or value of any scalar data-type, to variable, $new_var
In this example, value of variable, $new_var that is, array literal is assigned to a new key. */
echo '<pre>';
print_r( $new_var );
echo '</pre>'; // the OUTPUT is a NESTED ARRAY ?>
(
[0] => 3.14
[1] => Amit
[2] => Array
(
[0] => 3.14
[1] => Amit
)
)</pre>
The value of $new_var is already in RAM (temporary memory), and it is processed by the CPU, and assigned to a new key inside a variable name $new_var. You can easily access, or manipulate the (NESTED) items inside of an NESTED array, by same way. You have to place another (opening and closing) set of square brackets next to square brackets after the variable name, and so on...
<?php echo $new_var[2][0]; // first item from the NESTED array ?>
<?php echo $new_var[2][1]; // second item from the NESTED array ?>
<?php var_dump( $new_var[2] ); // you cannot access the NESTED array with echo
function ?>
Let's Create an Associative Array
<?php $assoc_var = []
; // No difference between indexed array or associative array ?>
<?php $assoc_var[] = 'one'; // new item added to indexed array ?>
<?php $assoc_var[] = 'two'; // another item added to indexed array ?>
<?php $assoc_var['a'] = 1; // new key and item added to array ?>
<?php $assoc_var['b'] = 2; // now indexed array is mixture of above keys and items ?>
<?php var_dump( $assoc_var ); // ?>
You can see in the above OUTPUT that $assoc_var has various keys for items inside it. These keys are 0, 1, 'a', and 'b'. When you don't provide any key when adding a new item to an array, the next greatest integer value will be automatically assigned as key (index number).
<?php $assoc_var[] = 'three'; // new item added to indexed array ?>
<?php $assoc_var[20] = 'four'; // new item added to index number, 20 ?>
<?php $assoc_var[] = 'five'; // new item added to indexed array ?>
<?php var_dump( $assoc_var ); // ?>
You can also create NESTED arrays of associative type, that is with string keys, etc. in the same manner as mentioned above.
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
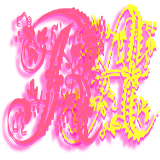
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇