
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Operator Precedence & Associativity in Category: PHP by amit
🕙 Posted on 2023-05-28 at 14:03:34 Read in Hindi ...
Comparison Operators
In previous page, you have seen difference between = (simple assignment) operator and == (equal to) operator. You have been also introduced with other comparison operators. Comparison operators, as their name implies, allow you to compare two values. In loose comparison (== equal to, and != not equal to), type juggling happens, that is when a number or numeric string is compared with other data-types, those are automatically type-casted to numeric data-type.
<?php
$abc = 5; $xyz = "5"; // variable $abc is integer, whereas $xyz is string.
var_dump( $abc ==
$xyz ); // equal to operator checks loose comparison, outputs bool(true)
echo '<br />';
var_dump( $abc ===
$xyz ); // identical to operator checks strict comparison, outputs bool(false)
echo '<br />';
var_dump( $abc !=
$xyz ); // not equal to operator checks loose comparison, outputs bool(false)
echo '<br />';
var_dump( $abc !==
$xyz ); // not identical to operator checks strict comparison, outputs bool(true)
echo '<br />';?>
=== (identical to) and !== (not identical to) operators compare not only the values of two literals (or variables/==
(equal to) operator loosely compares two literals or two variables/
<?php
var_dump( 5.5 == "5.5"
); // Outputs bool(true)
echo '<br />'; var_dump( null == ""
); // Outputs bool(true)
echo '<br />'; var_dump( false == ""
); // Outputs bool(true)
echo '<br />'; var_dump( 0 == false
); // Outputs bool(true)
echo '<br />'; var_dump( 0 == null
); // Outputs bool(true)
echo '<br />'; var_dump( 0 == "0"
); // Outputs bool(true)
echo '<br />'; var_dump( 7 == "07"
); // Outputs bool(true)
echo '<br />'; var_dump( 7 == "+07"
); // Outputs bool(true)
echo '<br />'; var_dump( 7 == " 07"
); // Outputs bool(true)
echo '<br />'; var_dump( 70 == 70.00
); // Outputs bool(true)
echo '<br />'; var_dump( 700 == "7e2"
); // Outputs bool(true)
echo '<br />'; var_dump( 2 == true
); // Outputs bool(true)
echo '<br />'; var_dump( "xyz" == true
); // Outputs bool(true)?>
The each of above example is known as 'FALSE POSITIVE' because all of these when loosely compared, output bool(true), but when they are strictly compared with ===
(identical to) operator, all of these will output bool(false). Any string that contains the letter E
(case insensitive) bounded by numbers will be seen as a number expressed in scientific notation. This can produce unexpected results, as described in above example.
Following four examples also output bool(true) before PHP 7.1.0 and neither E_NOTICE nor E_WARNING was raised. You will learn more about different types of ERRORS in later topics. In older versions of PHP, when a string data-type is loosely compared with integer or float data-types, the string is evaluated after type-juggling.
<?php var_dump( 0 == "" ); // Outputs bool(false) ?><br />
<?php var_dump( "0" == "" ); // Outputs bool(false) ?><br />
<?php var_dump( 0 == "abc" ); // Outputs bool(false) ?><br />
<?php var_dump( 3 == "3 sparrows" ); // Outputs bool(false) ?><br />
However, now in latest version of PHP, when a number is compared with a string data-type, and the string literal is not numeric string, then the number is type-casted into string data-type and then comparison happens. Therefore, you should carefully TEST and EXAMINE your PHP codes when hosting your CMS or WEBSITE on a SERVER having an older version of PHP. Other comparison operators are self-explanatory, and are described hereinbelow:
<?php var_dump( 5 < "6" ); // less than operator, outputs bool(true) ?><br />
<?php var_dump( 6 > "4" ); // greater than operator, outputs bool(true) ?><br />
<?php var_dump( 5 <= "6" ); // less than or equal to operator, outputs bool(true) ?><br />
<?php var_dump( 6 >= "4" ); // greater than or equal to operator, outputs bool(true) ?><br />
<?php var_dump( 6 <> 4 ); // less than or greater than (not equal to) operator, outputs bool(true) ?><br />
<?php var_dump( 6 <=> 4 ); // spaceship operator, outputs int(1) ?><br />
<?php var_dump( 6 <=> 6 ); // spaceship operator, outputs int(0) ?><br />
<?php var_dump( 4 <=> 6 ); // spaceship operator, outputs int(-1) ?><br />
<?php var_dump( [] == "" ); // Outputs bool(false) ?><br />
Operator Precedence & Associativity
Following table shows the HIGHER level of precedence of operators at the top, and LOWER level of precedence below these operators. It means that ** (exponent) operator has higher precedence over other operators. Remember basic arithmetic from school? These work just like those.
Arithmetic Operators
Example | Name | Result |
---|---|---|
+$a | Identity (unary) | Conversion of $a to int or float as appropriate. |
-$a | Negation (unary) | Opposite of $a. |
$a + $b | Addition | Sum of $a and $b. |
$a - $b | Subtraction | Difference of $a and $b. |
$a * $b | Multiplication | Product of $a and $b. |
$a / $b | Division | Quotient of $a and $b. |
$a % $b | Modulo | Remainder of $a divided by $b. |
$a ** $b | Exponentiation | Result of raising $a to the $b'th power. |
The unary + (identity) and - (negation) are prefix to any variable name or a literal. Also, ++ (increment) and -- (decrement) can be either prefix or postfix to any variable name or a literal. Increment and decrement operators are used to increase or decrease, respectively the value of a variable name by 1 (one).
Associativity | Operators | Additional Information |
---|---|---|
(n/a) | clone new | clone and new |
right | ** | arithmetic |
(n/a) | + - ++ -- ~ (int) (float) (string) (array) (object) (bool) @ | arithmetic (unary + and - ), increment / decrement, bitwise, type casting and error control |
left | instanceof | type |
(n/a) | ! | logical |
left | * / % | arithmetic |
left | + - . | arithmetic (binary + and - ), array and string (. prior to PHP 8.0.0) |
left | << >> | bitwise |
left | . | string (as of PHP 8.0.0) |
non-associative | < <= > >= | comparison |
non-associative | == != === !== <> <=> | comparison |
left | & | bitwise and references |
left | ^ | bitwise |
left | | | bitwise |
left | && | logical |
left | || | logical |
right | ?? | null coalescing |
non-associative | ? : | ternary(left-associative prior to PHP 8.0.0) |
right | = += -= *= **= /= .= %= &= |= ^= <<= >>= ??= | assignment |
(n/a) | yield from | yield from |
(n/a) | yield | yield |
(n/a) | print | |
left | and | logical |
left | xor | logical |
left | or | logical |
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
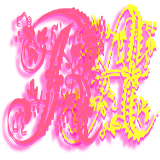
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇