
Please support if you like my work by payment through upi: sinhamit@icici or payment by bank
account name: Amit Kumar Sinha,
account number: 2646728782
IFSC code: KKBK0005660
SWIFT: KKBKINBB
Accessing Individual Elements From an Array in Category: PHP by amit
🕙 Posted on 2023-05-15 at 22:30:08 Read in Hindi ...
Accessing an item from an Array
You have various choices to do operations on array data-type. Consider the following example, of indexed array and its output with var_dump()
function. You have already seen in previous page, how keys are associated with their respective values, and also when the programmer doesn't provides specific key for an item, in an indexed array.
<?php $my_var = array
( "Amit", 'Krishna', true
, 1, 2.5 );
var_dump
( $my_var ); ?>
You can see in the above output, that index number for a key in an indexed array, starts from 0
(zero - first whole number). Therefore, you can access these items, by providing the key (either integer, or string) within the []
(opening and closing) square brackets just after the variable name or constant name.
<?php echo $my_var[0]; // first item of the array ?>
<?php echo $my_var[1]; // second item of the array ?>
Like above two lines of code, you can get the output of other items, when you already knew that how many items are in an array. The variable name $my_var has been assigned with the array literal, described in above example. But, when there are unknown number of items in an array, for example, when any topic or page is added in this website, a new item will automatically be added to an array, which will display all results properly.
Thus, when you will learn advanced programming techniques, it will be clear to you that a literal of any data-type cannot be directly assigned to a variable name or a constant name. Then, you have to use LOOPS, conditional operators, etc. to access data from the database table. Back to accessing items in an array, you have learned in previous page about count()
(pre-defined) function. It is used to get integer value of number of all items (elements) in an array.
Also, you have seen in the above output, that number of all items in an array, and number returned from count()
function is one more than the last index number of an indexed array. It is because the index number starts from 0
(zero), whereas the count()
function or the var_dump()
function outputs the number which starts from 1
. Therefore, to access the last item of an array, you have to subtract the number returned from count()
function minus one.
<?php echo $my_var[ count
( $my_var ) - 1 ]; // outputs last item of the array ?>
<?php echo $my_var[ count
( $my_var ) ]; // outputs ERROR, shown as below: ?>
Warning: Undefined array key 5 in C:\xampp\
In the topic String and String Functions, you have already seen that a single character can be accessed from a string literal in similar way. However, you must note that there is no negative index number for an indexed array. Therefore, unlike string data-type, you cannot access the last item from an array data-type, with -1 key. This will output ERROR as shown below:
<?php echo $my_var[ -1 ]; // outputs ERROR, shown as below: ?>
Warning: Undefined array key -1 in C:\xampp\
Accessing an item from an Associative Array
<?php $assoc_var = array
( 0 => "Amit", 1 => 'Krishna', 20 => true
, 3 => 1, 4 => 2.5 ); ?>
This variable, $assoc_var is assigned with an associative array literal, whose values in respective keys are exactly same as those values in above variable, $my_var. But there is a fine difference between the two. The third item in $assoc_var is associated with 20
key, instead of 2. You cannot access an item from an associative array, whose key does not exist. You should visit the previous page, if you want to know how to create an associative array.
<?php echo $assoc_var[20]; // item at index 20 of the array ?>
Whether the key is of string data-type, or integer data-type, you should carefully name the columns of a table, in the database. The key in the square brackets just after the variable name, must be exactly same as that key mentioned in the associative array. If the key is of string data-type, you must enclose it within a pair of single quotes or a pair of double quotes.
Let't create an Associative Array with string keys
<?php $string_assoc = array
( 'zero' => "Amit", 'one' => 'Krishna', 'two' => true
, 'three' => 1, 'four' => 2.5 );
echo '<pre>';
var_dump( $string_assoc );
echo '</pre>'; ?><br />
["zero"]=>
string(4) "Amit"
["one"]=>
string(7) "Krishna"
["two"]=>
bool(true)
["three"]=>
int(1)
["four"]=>
float(2.5)
}
</pre><br />
The keys, "zero", "one", "two", etc. are not equivalent to 0, 1, 2, and so on... Even a single character, for example, "a" or "z" can be name of the keys inside the parentheses of array()
or []
for an item. In JavaScript Object data-type, keys can be without a pair of single quotes or a pair of double quotes, and those will be valid key name. But in PHP, you have to enclose every string data-type, with a pair of single/
<?php echo $string_assoc[ "zero" ]; // the string key cannot be said as for first item ?><br />
<?php echo $string_assoc[ "one" ]; // there is no numeric key in this array ?><br />
Indentation is not important in PHP, and following example of an associative array can be either written in one line, or can be written beautifully with indentation.
<?php
$user_data = array
(
'first_name' => "Amit",
'last_name' => 'Sinha',
'age' => 42,
'gender' => "male",
'nationality' => "Indian",
'Address' => "Not to be disclosed"
);
echo '<pre>';
var_dump( $user_data );
echo '</pre>'; ?>
["first_name"]=>
string(4) "Amit"
["last_name"]=>
string(5) "Sinha"
["age"]=>
int(42)
["gender"]=>
string(4) "male"
["nationality"]=>
string(6) "Indian"
["Address"]=>
string(19) "Not to be disclosed"
}
</pre>
You can access individual items from the above mentioned array, in following ways:
<?php echo $user_data[ "first_name" ]; ?>
<?php echo $user_data[ "last_name" ]; ?>
Leave a Comment:

Amit Sinha March 2nd, 2023 at 9:30 PM
😃 😄 😁 😆 😅 😂 😉 😊 😇 😍 😘 😚 😋 😜 😝 😶 😏 😒 😌 😔 😪 😷 😵 😎 😲 😳 😨 😰 😥 😢 😭 😱 😖 😣 😞 😓 😩 😫 😤
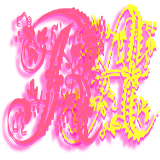
Ribhu March 3rd, 2023 at 9:30 PM
🐵 🐒 🐶 🐩 🐺 🐱 🐯 🐅 🐆 🐴 🐎 🐮 🐂 🐃 🐄 🐷 🐖 🐗 🐽 🐏 🐑 🐐 🐪 🐫 🐘 🐭 🐁 🐀 🐹 🐰 🐇